[UPDATED] Clipboard Functions (that WORK) |
Author: |
Message: |
deAd
Scripting Contest Winner
    

Posts: 1060 Reputation: 28
– / / 
Joined: Jan 2006
|
O.P. [UPDATED] Clipboard Functions (that WORK)
UPDATED: If you have added the readClipboard() function to your script, update it now. Fixed major bug that caused the clipboard to stay locked on certain occasions.
Here's some great functions I made to get the clipboard text and change the clipboard text! They are based off the non-functional code in these requests: Get Text and Set Text. They are fully functional, but BE CAREFUL when you modify them. I haven't had any problems yet.
Be careful when you modify these.
@Segosa - your problem was (1) no unicode and (2) you did it a bit wrong
Clear Text:
No return value.
code: function clearClipboard(){
if (Interop.Call("User32", "OpenClipboard", 0)){
Interop.Call("User32", "EmptyClipboard");
Interop.Call("User32", "CloseClipboard");
}
}
Get Text:
Do not remove the try/catch statement. Your clipboard may lock up.
This function will return false if there are errors (including the text being too long. I don't know how long it can go.) or if the clipboard is currently in use by another program. Otherwise it will return the text
code: function readClipboard() {
var CF_TEXT = 1;
var CF_OEMTEXT = 7;
var CF_UNICODETEXT = 13;
var toBeReturned = false;
try {
if (Interop.Call("User32", "OpenClipboard", 0)) {
if (Interop.Call("User32", "IsClipboardFormatAvailable", CF_TEXT | CF_OEMTEXT)) {
var hClipboardData = Interop.Call("User32", "GetClipboardData", CF_UNICODETEXT);
var pchData = Interop.Call("Kernel32", "GlobalLock", hClipboardData);
var size = Interop.Call("Kernel32", "GlobalSize", hClipboardData);
var str = Interop.Allocate(size+2);
Interop.Call("Kernel32", "RtlMoveMemory", str, pchData, size);
toBeReturned = str.ReadString(0);
var unlocked = Interop.Call("Kernel32", "GlobalUnlock", hClipboardData);
} else {
Interop.Call("User32", "CloseClipboard");
return false;
}
Interop.Call("User32", "CloseClipboard");
}
} catch(ex) {
Interop.Call("User32", "CloseClipboard");
return false;
}
return toBeReturned;
}
Set Text:
This function has no return value. To use it, type writeClipboard(String);.
code: function writeClipboard(str){
var size = str.length*2+2;
var GMEM_MOVEABLE = 0x2;
var GMEM_SHARE = 0x2000;
var GMEM_ZEROINIT = 0x40;
var memtype = GMEM_MOVEABLE | GMEM_SHARE | GMEM_ZEROINIT;
if (Interop.Call("User32", "OpenClipboard", 0)){
var heap = Interop.Call("Kernel32", "GlobalAlloc", 0, size);
var p = Interop.Call("Kernel32", "GlobalLock", heap);
Interop.Call('Kernel32','RtlMoveMemory',p,str,size);
Interop.Call("Kernel32", "GlobalUnlock", heap);
if (Interop.Call("User32", "OpenClipboard", 0)){
Interop.Call("User32", "EmptyClipboard");
Interop.Call("User32", "SetClipboardData", 13, heap);
Interop.Call("User32", "CloseClipboard");
}
}
}
This post was edited on 07-12-2006 at 01:16 PM by deAd.
|
|
07-11-2006 09:27 PM |
|
 |
andrey
elite shoutboxer
   

Posts: 793 Reputation: 48
– / / 
Joined: Aug 2004
|
RE: Clipboard Functions (that WORK)
wow,
i would have never figured that out myself, thank you!
do we actually have some kind of database for these code pieces?
would definately be useful to get them into one place..
uh..one thing I just noticed with the get Text code:
if the text in the clipboard is longer than 23 chars, it returns 'false'
if the text in the clipboard is longer than 23 chars, it returns code: Interop.Call failed to locate function "char23char24char25etc"
False
This post was edited on 07-11-2006 at 10:00 PM by andrey.
|
|
07-11-2006 09:44 PM |
|
 |
deAd
Scripting Contest Winner
    

Posts: 1060 Reputation: 28
– / / 
Joined: Jan 2006
|
O.P. RE: Clipboard Functions (that WORK)
quote: Originally posted by andrey
uh..one thing I just noticed with the get Text code:
if the text in the clipboard is longer than 23 chars, it returns 'false'
if the text in the clipboard is longer than 23 chars, it returnscode: Interop.Call failed to locate function "char23char24char25etc"
False
I got this when I started making it, but it went away. It works fine now, so I don't understand what's wrong.
About the database, there is no official one, but there is always http://www.mpwiki.net 
This post was edited on 07-11-2006 at 10:07 PM by deAd.
|
|
07-11-2006 09:46 PM |
|
 |
ShawnZ
Veteran Member
    

Posts: 3141 Reputation: 43
33 / / 
Joined: Jan 2003
|
RE: Clipboard Functions (that WORK)
what if the clipboard data isn't a string?
Spoiler: the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
the game.
|
|
07-11-2006 10:45 PM |
|
 |
deAd
Scripting Contest Winner
    

Posts: 1060 Reputation: 28
– / / 
Joined: Jan 2006
|
O.P. RE: Clipboard Functions (that WORK)
The functions here deal with strings only. readClipboard() will return false if the data is not a string.
This post was edited on 07-11-2006 at 11:00 PM by deAd.
|
|
07-11-2006 10:56 PM |
|
 |
alexp2_ad
Scripting Contest Winner
   
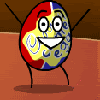
Who love the chocolate?
Posts: 691 Reputation: 26
37 / / –
Joined: May 2004
Status: Away
|
RE: [UPDATED] Clipboard Functions (that WORK)
Very nice work!
Been wondering about this. 
|
|
07-12-2006 12:32 AM |
|
 |
andrey
elite shoutboxer
   

Posts: 793 Reputation: 48
– / / 
Joined: Aug 2004
|
RE: [UPDATED] Clipboard Functions (that WORK)
hmm..there's still something wrong with this, at some occasions my clipboard is still not getting closed again
save this as a new script:
code: //ABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZ <-- for testing
Interop.Call("user32", "CloseClipboard");
Debug.Trace(readClipboard());
function readClipboard() {
var CF_TEXT = 1;
var CF_OEMTEXT = 7;
var CF_UNICODETEXT = 13;
try {
if (Interop.Call("User32", "OpenClipboard", 0)) {
if (Interop.Call("User32", "IsClipboardFormatAvailable", CF_TEXT | CF_OEMTEXT)) {
var hClipboardData = Interop.Call("User32", "GetClipboardData", CF_UNICODETEXT);
var pchData = Interop.Call("Kernel32", "GlobalLock", hClipboardData);
var size = Interop.Call("Kernel32", "GlobalSize", hClipboardData);
var str = "";
Interop.Call("Kernel32", "RtlMoveMemory", str, pchData, size);
var unlocked = Interop.Call("Kernel32", "GlobalUnlock", hClipboardData);
} else {
Interop.Call("User32", "CloseClipboard");
return false;
}
Interop.Call("User32", "CloseClipboard");
}
} catch(ex) {
Interop.Call("User32", "CloseClipboard");
return false;
}
return str;
}
now copy (to the clipboard)
ABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZ
and save the script, everything works fine and we get a 'False' quote: Originally posted by Script Debugging
Script has been stopped
Script is starting
Interop.Call failed to locate function "YZABCDEFGHIJKLMNOPQRSTUVWXYZ"
False
Script is now loaded and ready
but now delete the 3rd row
"Interop.Call("user32", "CloseClipboard");"
or put it as comment, again copy
ABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZ
and save the script. Now we get an error quote: Originally posted by Script Debugging
Script has been stopped
Script is starting
Interop.Call failed to locate function "YZABCDEFGHIJKLMNOPQRSTUVWXYZ"
Interop.Call failed to locate function "QRSTUVWXYZ"
Error: .
Line: 26. Code: -2147467259.
Script has failed to start
the script fails, and the clipboard isn't closed.
Edit:
Thanks dt, that worked.
deAd, please update your post with dt's code
This post was edited on 07-12-2006 at 12:02 PM by andrey.
|
|
07-12-2006 02:58 AM |
|
 |
-dt-
Scripting Contest Winner
    

;o
Posts: 1818 Reputation: 74
36 / / 
Joined: Mar 2004
|
RE: [UPDATED] Clipboard Functions (that WORK)
try this
code: function readClipboard() {
var CF_TEXT = 1;
var CF_OEMTEXT = 7;
var CF_UNICODETEXT = 13;
var toBeReturned = false;
try {
if (Interop.Call("User32", "OpenClipboard", 0)) {
if (Interop.Call("User32", "IsClipboardFormatAvailable", CF_TEXT | CF_OEMTEXT)) {
var hClipboardData = Interop.Call("User32", "GetClipboardData", CF_UNICODETEXT);
var pchData = Interop.Call("Kernel32", "GlobalLock", hClipboardData);
var size = Interop.Call("Kernel32", "GlobalSize", hClipboardData);
var str = Interop.Allocate(size+2);
Interop.Call("Kernel32", "RtlMoveMemory", str, pchData, size);
toBeReturned = str.ReadString(0);
var unlocked = Interop.Call("Kernel32", "GlobalUnlock", hClipboardData);
} else {
Interop.Call("User32", "CloseClipboard");
return false;
}
Interop.Call("User32", "CloseClipboard");
}
} catch(ex) {
Interop.Call("User32", "CloseClipboard");
return false;
}
return toBeReturned;
}
Happy Birthday, WDZ
|
|
07-12-2006 04:45 AM |
|
 |
Dempsey
Scripting Contest Winner
    

http://AdamDempsey.net
Posts: 2395 Reputation: 53
38 / / 
Joined: Jul 2003
|
RE: [UPDATED] Clipboard Functions (that WORK)
quote: Originally posted by andrey
do we actually have some kind of database for these code pieces?
would definately be useful to get them into one place..
There is MPWiki and the soon to be released MPScripts which has a code snippet database.
|
|
07-12-2006 07:28 AM |
|
 |
|
|