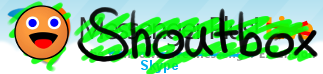
Memory Useage In VB - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Skype & Technology (/forumdisplay.php?fid=9)
+---- Forum: Tech Talk (/forumdisplay.php?fid=17)
+----- Thread: Memory Useage In VB (/showthread.php?tid=30654)
Memory Useage In VB by DJeX on 08-29-2004 at 07:17 AM
I'm making a program in VB that will display the netstats of your computer. Every time it updates it stores the information in memory. Which every 5 seconds raises the program memery useage by 24bytes. I no it may seem like not much but when this program is ment to run when you start your computer and run in the background, 24 bytes adds up. So I need to know how to clear the memory of the information I've stored in it. Heres my code that adds the info in the memory.
code: Public Sub RefreshStack()
Dim i As Long
pDataRef = 0
For i = 0 To nRows '// read 24 bytes at a time
CopyMemory nState, ByVal pTablePtr + (pDataRef + 4), 4
CopyMemory nLocalAddr, ByVal pTablePtr + (pDataRef + 8), 4
CopyMemory nLocalPort, ByVal pTablePtr + (pDataRef + 12), 4
CopyMemory nRemoteAddr, ByVal pTablePtr + (pDataRef + 16), 4
CopyMemory nRemotePort, ByVal pTablePtr + (pDataRef + 20), 4
CopyMemory nProcId, ByVal pTablePtr + (pDataRef + 24), 4
DoEvents
'If nRemoteAddr <> 0 Or nRemotePort <> 0 Or nLocalPort <> 0 Then
Connection .State = nState
Connection .LocalHost = nLocalAddr
Connection .LocalPort = nLocalPort
Connection .RemoteHost = nRemoteAddr
Connection .RemotePort = nRemotePort
Connection .ProcessID = nProcId
Connection .ProcessName = GetProcessName(nProcId)
'End If
pDataRef = pDataRef + 24
DoEvents
Next i
End Sub
Now how do I clear or remove the data so I can keep my memory free for other programs to use. And so it don't hog all the memory.
Thanks!
RE: Memory Useage In VB by theRabbit83 on 08-29-2004 at 08:28 AM
Are you sure that code keeps adding up memory usage? I may be wrong but it seems that you are storing your info on the connection vector, and each time you are reusing it by going trough it from the 0 position to nRows... what memory (from wich variables) do you want to free?
RE: Memory Useage In VB by DJeX on 08-29-2004 at 07:40 PM
No it adds 24 bytes ever time it udates. I can even see it when i go into the task manager and look at the programs mem useage.
RE: Memory Useage In VB by Choli on 08-29-2004 at 08:58 PM
quote: Originally posted by DJeX
it adds 24 bytes ever time it udates. I can even see it when i go into the task manager and look at the programs mem useage.
first of all: The values of memory usage shown in the task manager doesn't represent the real memory used by the programs, so don't worry very much about it. Are you sure it adds 24 bytes every update and forever? or does it stop at some value?
Well, about the code, I don't see anything wrong, however you don't posted all the code (in fact, I'm more interested in all the declarations of those variables and functions). First of all: Are you sure you need the calls to copymemory? i don't know where those pTablePtr and pDataRef come from, however maybe you could use a direct assignment.
And second thing: Again this is a supposition (because i don't know the declaration of the variables). What about using VarPrt? I mean:
CopyMemory VarPrt(nState), ByVal pTablePtr + (pDataRef + 4), 4
CopyMemory VarPrt(nLocalAddr), ByVal pTablePtr + (pDataRef + 8), 4
etc...
Well, if the code works, I suppose it's fine as you wrote it. (Also, at the first CopyMemory call, is it "pDataRef + 4" or just pDataRef? (and similar for other calls))
RE: Memory Useage In VB by DJeX on 08-29-2004 at 09:04 PM
my whole modual
code: Option Explicit
Type MIB_UDPROW
dwLocalAddr As String * 4 'address on local computer
dwLocalPort As String * 4 'port number on local computer
End Type
'Private Type MIB_TCPROW
' dwState As Long
' dwLocalAddr As Long
' dwLocalPort As Long
' dwRemoteAddr As Long
' dwRemotePort As Long
'End Type
Type MIB_TCPROW
dwState As Long 'state of the connection
dwLocalAddr As String * 4 'address on local computer
dwLocalPort As String * 4 'port number on local computer
dwRemoteAddr As String * 4 'address on remote computer
dwRemotePort As String * 4 'port number on remote computer
End Type
Private Declare Function GetProcessHeap Lib "kernel32" () As Long
Private Declare Function htons Lib "ws2_32.dll" (ByVal dwLong As Long) As Long
Public Declare Function AllocateAndGetTcpExTableFromStack Lib "iphlpapi.dll" (pTcpTableEx As Any, ByVal bOrder As Long, ByVal heap As Long, ByVal zero As Long, ByVal Flags As Long) As Long
Public Declare Function AllocateAndGetUdpExTableFromStack Lib "iphlpapi.dll" (pUdpTableEx As Any, ByVal bOrder As Long, ByVal heap As Long, ByVal zero As Long, ByVal Flags As Long) As Long
Private Declare Function SetTcpEntry Lib "iphlpapi.dll" (pTcpTableEx As MIB_TCPROW) As Long
Public Declare Sub CopyMemory Lib "kernel32" Alias "RtlMoveMemory" (Destination As Any, Source As Any, ByVal Length As Long)
Private pTablePtr As Long
Private pDataRef As Long
Public nRows As Long
Public oRows As Long
Private nCurrentRow As Long
Private udtRow As MIB_TCPROW
Private nState As Long
Private nLocalAddr As Long
Private nLocalPort As Long
Private nLocalAddr2 As Long
Private nLocalPort2 As Long
Private nRemoteAddr As Long
Private nRemotePort As Long
Private nProcId As Long
Private nProcName As String
Public nRet As Long
Private Type Connection_
FileName As String
ProcessID As Long
ProcessName As String
LocalPort As Long
RemotePort As Long
LocalHost As Long
RemoteHost As Long
State As String
End Type
Public Connection(2000) As Connection_
Public Function GetIPAddress(dwAddr As Long) As String
Dim arrIpParts(3) As Byte
CopyMemory arrIpParts(0), dwAddr, 4
GetIPAddress = CStr(arrIpParts(0)) & "." & _
CStr(arrIpParts(1)) & "." & _
CStr(arrIpParts(2)) & "." & _
CStr(arrIpParts(3))
End Function
Public Function GetPort(ByVal dwPort As Long) As Long
GetPort = htons(dwPort)
End Function
Public Sub RefreshStack()
Dim i As Long
pDataRef = 0
For i = 0 To nRows '// read 24 bytes at a time
CopyMemory nState, ByVal pTablePtr + (pDataRef + 4), 4
CopyMemory nLocalAddr, ByVal pTablePtr + (pDataRef + 8), 4
CopyMemory nLocalPort, ByVal pTablePtr + (pDataRef + 12), 4
CopyMemory nRemoteAddr, ByVal pTablePtr + (pDataRef + 16), 4
CopyMemory nRemotePort, ByVal pTablePtr + (pDataRef + 20), 4
CopyMemory nProcId, ByVal pTablePtr + (pDataRef + 24), 4
DoEvents
'If nRemoteAddr <> 0 Or nRemotePort <> 0 Or nLocalPort <> 0 Then
Connection .State = nState
Connection .LocalHost = nLocalAddr
Connection .LocalPort = nLocalPort
Connection .RemoteHost = nRemoteAddr
Connection .RemotePort = nRemotePort
Connection .ProcessID = nProcId
Connection .ProcessName = GetProcessName(nProcId)
'End If
pDataRef = pDataRef + 24
DoEvents
Next i
End Sub
Public Function GetEntryCount() As Long
GetEntryCount = nRows - 1 '// The last entry is always an EOF of sorts
End Function
Public Function TerminateThisConnection(rNum As Long) As Boolean
On Error GoTo ErrorTrap
udtRow.dwLocalAddr = Connection(rNum).LocalHost
udtRow.dwLocalPort = Connection(rNum).LocalPort
udtRow.dwRemoteAddr = Connection(rNum).RemoteHost
udtRow.dwRemotePort = Connection(rNum).RemotePort
udtRow.dwState = 12
SetTcpEntry udtRow
TerminateThisConnection = True
Exit Function
ErrorTrap:
TerminateThisConnection = False
End Function
Public Function GetRefreshTCP() As Boolean
nRet = AllocateAndGetTcpExTableFromStack(pTablePtr, 0, GetProcessHeap, 0, 2)
If nRet = 0 Then
CopyMemory nRows, ByVal pTablePtr, 4
Else
GetRefreshTCP = False
Exit Function
End If
If nRows = 0 Or pTablePtr = 0 Then
GetRefreshTCP = False
Exit Function
End If
If oRows <> nRows Then
GetRefreshTCP = True
Else
GetRefreshTCP = False
End If
oRows = nRows
End Function
Function d_state(s) As String
Select Case s
Case "0": d_state = "UNKNOWN"
Case "1": d_state = "CLOSED"
Case "2": d_state = "LISTENING"
Case "3": d_state = "SYN_SENT"
Case "4": d_state = "SYN_RCVD"
Case "5": d_state = "ESTABLISHED"
Case "6": d_state = "FIN_WAIT1"
Case "7": d_state = "FIN_WAIT2"
Case "8": d_state = "CLOSE_WAIT"
Case "9": d_state = "CLOSING"
Case "10": d_state = "LAST_ACK"
Case "11": d_state = "TIME_WAIT"
Case "12": d_state = "DELETE_TCB"
End Select
End Function
RE: Memory Useage In VB by Choli on 08-29-2004 at 09:23 PM
quote: Originally posted by DJeX
nRet = AllocateAndGetTcpExTableFromStack(pTablePtr, 0, GetProcessHeap, 0, 2)
Well, I don't know what that function does (in fact now it's the first time that I see it), anyway, I think (reading its name) that it allocates (ie: creates, takes, ...) some memory. That means that once you've called AllocateAndGetTcpExTableFromStack, your program has some more memory used. If after using the table it creates (ie: the pTablePtr) you don't free that memory, it'll sty there forever. So: you call AllocateAndGetTcpExTableFromStack, use your RefreshStack function and then you have to free that memory using some API. Which one? Well, i don't know, but I'm sure it's inside the iphlpapi.dll library. you'll have to search it
RE: Memory Useage In VB by DJeX on 08-29-2004 at 11:59 PM
What if I tryed to use this:
code: Private Declare Function LocalFree Lib "kernel32" (ByVal hMem As Long) As Long
Public Sub FreeMemory()
LocalFree pTablePtr
End Sub
Would that work?
RE: Memory Useage In VB by Choli on 08-30-2004 at 09:23 PM
quote: Originally posted by DJeX
Would that work?
I don't know. Maybe. Note that when you pass the pointer pTablePtr (just allocated by AllocateAndGetTcpExTableFromStack) to LocalFree, that API (LocalFree) has to know how much memory it has to free, and it will only be able to know that if the memory was allocated by another API "relative" to it. I mean, if AllocateAndGetTcpExTableFromStack calls internally some API that LocalFree knows, then yes, you'll be able to use it else you can't.
Anyway, I've searched a bit, and I've found this. Try it. It looks nice, so I think it'll work, but i don't know:
code: Declare Function HeapFree Lib "kernel32" Alias "HeapFree" (ByVal hHeap As Long, ByVal dwFlags As Long, lpMem As Any) As Long
Declare Function GetProcessHeap Lib "kernel32" Alias "GetProcessHeap" () As Long
public sub freemem()
HeapFree GetProcessHeap(), 0&, ByVal pTablePtr
' if it doesn't work, try to remove the ByVal keyword
end sub
RE: Memory Useage In VB by DJeX on 09-01-2004 at 05:17 AM
Works Great! Thanks!
RE: Memory Useage In VB by Choli on 09-01-2004 at 08:02 PM
quote: Originally posted by DJeX
Works Great! Thanks!
no problem 
quote: Originally posted by Choli
HeapFree GetProcessHeap(), 0&, ByVal pTablePtr
' if it doesn't work, try to remove the ByVal keyword
does it work like I posted or did you remove the ByVal?
RE: Memory Useage In VB by DJeX on 09-02-2004 at 03:48 AM
It doesent make a diffrence.
|