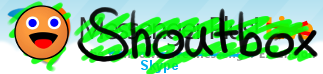
VBScript & coding tips - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Skype & Technology (/forumdisplay.php?fid=9)
+---- Forum: Tech Talk (/forumdisplay.php?fid=17)
+----- Thread: VBScript & coding tips (/showthread.php?tid=31208)
VBScript & coding tips by CookieRevised on 09-10-2004 at 07:03 AM
[Regular Expressions]
quote: Originally posted by |\/|artij|\| in this thread
Regular Expressions in the replace function, TB told me you could only do that in JavaScript. Cookie, you'd do me a very big favor if you could provide a good example of that, thanks.
Related collections and objects for vbscript:
RegExp Object (vbscript 5+):
Properties:
* Global
* IgnoreCase
* Pattern
Methods:
* Execute
* Replace
* Test
Match Object (vbscript 5+):
Properties:
* FirstIndex
* Length
* Value
Matches Collection (vbscript 1+)
SubMatches Collection (vbscript 5.5+)
Example 1:code: Function RegExpTest(patrn, strng)
Dim regEx, Match, Matches ' Create variable.
Set regEx = New RegExp ' Create a regular expression.
regEx.Pattern = patrn ' Set pattern.
regEx.IgnoreCase = True ' Set case insensitivity.
regEx.Global = True ' Set global applicability.
Set Matches = regEx.Execute(strng) ' Execute search.
For Each Match in Matches ' Iterate Matches collection.
RetStr = RetStr & "Match found at position "
RetStr = RetStr & Match.FirstIndex & ". Match Value is '"
RetStr = RetStr & Match.Value & "'." & vbCRLF
Next
RegExpTest = RetStr
End Function
MsgBox(RegExpTest("is.", "IS1 is2 IS3 is4"))
Example 2:code: Function RegExpTest(patrn, strng)
Dim regEx, retVal ' Create variable.
Set regEx = New RegExp ' Create regular expression.
regEx.Pattern = patrn ' Set pattern.
regEx.IgnoreCase = False ' Set case sensitivity.
retVal = regEx.Test(strng) ' Execute the search test.
If retVal Then
RegExpTest = "One or more matches were found."
Else
RegExpTest = "No match was found."
End If
End Function
MsgBox(RegExpTest("is.", "IS1 is2 IS3 is4"))
Example 3:code: Function ReplaceTest(patrn, replStr)
Dim regEx, str1 ' Create variables.
str1 = "The quick brown fox jumped over the lazy dog."
Set regEx = New RegExp ' Create regular expression.
regEx.Pattern = patrn ' Set pattern.
regEx.IgnoreCase = True ' Make case insensitive.
ReplaceTest = regEx.Replace(str1, replStr) ' Make replacement.
End Function
MsgBox(ReplaceTest("fox", "cat")) ' Ex1: Replace 'fox' with 'cat'.
MsgBox(ReplaceText("(\S+)(\s+)(\S+)", "$3$2$1")) ' Ex2: Swap pairs of words.
Example 4:code: Function SubMatchTest(inpStr)
Dim oRe, oMatch, oMatches
Set oRe = New RegExp
' Look for an e-mail address (not a perfect RegExp)
oRe.Pattern = "(\w+)@(\w+)\.(\w+)"
' Get the Matches collection
Set oMatches = oRe.Execute(inpStr)
' Get the first item in the Matches collection
Set oMatch = oMatches(0)
' Create the results string.
' The Match object is the entire match - dragon@xyzzy.com
retStr = "Email address is: " & oMatch & vbNewline
' Get the sub-matched parts of the address.
retStr = retStr & "Email alias is: " & oMatch.SubMatches(0) ' dragon
retStr = retStr & vbNewline
retStr = retStr & "Organization is: " & oMatch. SubMatches(1)' xyzzy
SubMatchTest = retStr
End Function
MsgBox(SubMatchTest("Please send mail to dragon@xyzzy.com. Thanks!"))
[Replace Function]
(vbscript 2+)
I've seen in some of the Stuffplug-NG VBS talkers that replacements are done like so:code: ...some code...
output = Replace(output, "some text", "blah")
output = Replace(output, "Some Text", "blah")
output = Replace(output, "SOME TEXT", "blah")
...some code...
This isn't needed and can be done much shorter and thus much quicker, because the syntax of the function is:
Replace(expression, find, replacewith[, start[, count[, compare]]])
where
expression: Required. String expression containing substring to replace.
find: Required. Substring being searched for.
replacewith: Required. Replacement substring.
start: Optional. Position within expression where substring search is to begin. If omitted, 1 is assumed. Must be used in conjunction with count.
count: Optional. Number of substring substitutions to perform. If omitted, the default value is -1, which means make all possible substitutions. Must be used in conjunction with start.
compare: Optional. Numeric value indicating the kind of comparison to use when evaluating substrings. If omitted, the default value is 0, which means perform a binary comparison. 1 means a textual comparison (case insensitive). Constant vbBinaryCompare equals 0, and constant vbTextCompare equals 1.
Example 1:
code: Dim MyString
' A binary comparison starting at the beginning of the string. Returns "XXYXXPXXY".
MyString = Replace("XXpXXPXXp", "p", "Y")
' A textual comparison starting at the beginning of the string. Returns "XXYXXYXXY".
MyString = Replace("XXpXXPXXp", "p", "Y",,, 1)
' A textual comparison starting at position 4. Returns "XXYXXY".
MyString = Replace("XXpXXPXXp", "p", "Y", 4, -1, 1)
Example 2:
code: ...some code...
output = Replace(output, "some text", "blah",,, 1)
...some code...
Note:
Same syntax is used in Visual Basic and same principal goes for coding in other languages as well...
[Notes]
More examples and info on vbscript in various (official) sources on the net and in various (official) helpfiles...
The following lists the versions of Microsoft Visual Basic Scripting Edition implemented by host applications:
Microsoft Internet Explorer 3.0 => vbscript 1
Microsoft Internet Information Server 3.0 => vbscript 2
Microsoft Internet Explorer 4.0 => vbscript 3
Microsoft Internet Information Server 4.0 => vbscript 3
Microsoft Windows Scripting Host 1.0 => vbscript 3
Microsoft Outlook 98 => vbscript 3
Microsoft Visual Studio 6.0 => vbscript 4
Microsoft Internet Explorer 5.0 => vbscript 5
Microsoft Internet Information Services 5.0 => vbscript 5
Microsoft Internet Explorer 5.5 => vbscript 5.5
Microsoft Visual Studio .NET => vbscript 5.6
http://msdn.microsoft.com/library/default.asp?url.../html/vbstutor.asp
|