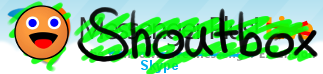
Help with Visual Basic code - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Skype & Technology (/forumdisplay.php?fid=9)
+---- Forum: Tech Talk (/forumdisplay.php?fid=17)
+----- Thread: Help with Visual Basic code (/showthread.php?tid=37219)
Help with Visual Basic code by DJeX on 01-19-2005 at 11:06 PM
I found this code on PlanetSourceCode. But would like to change it a bit.
Heres the code:
code: Option Compare Text
Option Explicit
#Const DebugMode = 1 ' whether or not to show debug information
'@===========================================================================
' SaveFormState:
' Saves the state of controls to a file
'
' Currently Supports: TextBox, CheckBox, OptionButton, Listbox, ComboBox
'=============================================================================
Sub SaveFormState(ByVal SourceForm As Form)
Dim A As Long ' general purpose
Dim B As Long
Dim C As Long
Dim FileName As String ' where to save to
Dim FHandle As Long ' FileHandle
' error handling code
On Error GoTo fError
' we create a filename based on the formname
FileName = App.Path + "\" + SourceForm.Name + ".set"
' Get a filehandle
FHandle = FreeFile()
' open the file
#If DebugMode = 1 Then
Debug.Print "--------------------------------------------------------->"
Debug.Print "Saving Form State:" + SourceForm.Name
Debug.Print "FileName=" + FileName
#End If
Open FileName For Output As FHandle
' loop through all controls
' first we save the type then the name
For A = 0 To SourceForm.Controls.Count - 1
#If DebugMode = 1 Then
Debug.Print "Saving control:" + SourceForm.Controls .Name
#End If
' if its textbox we save the .text property
If TypeOf SourceForm.Controls Is TextBox Then
Print #FHandle, "TextBox"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, "StartText"
Print #FHandle, SourceForm.Controls .Text
Print #FHandle, "EndText"
Print #FHandle, SourceForm.Controls .BackColor
' print a separator
Print #FHandle, "|<->|"
End If
' if its a checkbox we save the .value property
If TypeOf SourceForm.Controls Is CheckBox Then
Print #FHandle, "CheckBox"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, Str(SourceForm.Controls .Value)
' print a separator
Print #FHandle, "|<->|"
End If
' if its a option button we save its value
If TypeOf SourceForm.Controls Is OptionButton Then
Print #FHandle, "OptionButton"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, Str(SourceForm.Controls .Value)
' print a separator
Print #FHandle, "|<->|"
End If
' if its a listbox we save the .text and list contents
If TypeOf SourceForm.Controls Is ListBox Then
Print #FHandle, "ListBox"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, SourceForm.Controls .Text
Print #FHandle, "StartList"
For B = 0 To SourceForm.Controls .ListCount - 1
Print #FHandle, SourceForm.Controls .List
Next B
Print #FHandle, "EndList"
' save listindex
Print #FHandle, CStr(SourceForm.Controls .ListIndex)
' print a separator
Print #FHandle, "|<->|"
End If
' if its a combobox, save .text and list items
If TypeOf SourceForm.Controls Is ComboBox Then
Print #FHandle, "ComboBox"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, SourceForm.Controls .Text
Print #FHandle, "StartList"
For B = 0 To SourceForm.Controls .ListCount - 1
Print #FHandle, SourceForm.Controls .List
Next B
Print #FHandle, "EndList"
' print a separator
Print #FHandle, "|<->|"
End If
Next A
' close file
#If DebugMode = 1 Then
Debug.Print "Closing File."
Debug.Print "<----------------------------------------------------------"
#End If
Close #FHandle
' stop error handler
On Error GoTo 0
Exit Sub
fError: ' Simple error handler
C = MsgBox("Error in SaveFormState. " + Err.Description + ", Number=" + CStr(Err.Number), vbAbortRetryIgnore)
If C = vbIgnore Then Resume Next
If C = vbRetry Then Resume
' else abort
End Sub
'@===========================================================================
' LoadFormState:
' Loads the state of controls from file
'
' Currently Supports: TextBox, CheckBox, OptionButton, Listbox, ComboBox
'=============================================================================
Sub LoadFormState(ByVal SourceForm As Form)
Dim A As Long ' general purpose
Dim B As Long
Dim C As Long
Dim TXT As String ' general purpose
Dim fData As String ' used to hold File Data
' these are variables used for controls data
Dim cType As String ' Type of control
Dim cName As String ' Name of control
Dim cNum As Integer ' number of control
' vars for the file
Dim FileName As String ' where to save to
Dim FHandle As Long ' FileHandle
' error handling code
'On Error GoTo fError
' we create a filename based on the formname
FileName = App.Path + "\" + SourceForm.Name + ".set"
' abort if file does not exist
If Dir(FileName) = "" Then
#If DebugMode = 1 Then
Debug.Print "File Not found:" + FileName
#End If
Exit Sub
End If
' Get a filehandle
FHandle = FreeFile()
' open the file
#If DebugMode = 1 Then
Debug.Print "------------------------------------------------------>"
Debug.Print "Loading FormState:" + SourceForm.Name
Debug.Print "FileName:" + FileName
#End If
Open FileName For Input As FHandle
' go through file
While Not EOF(FHandle)
Line Input #FHandle, cType
Line Input #FHandle, cName
' Get control number
cNum = -1
For A = 0 To SourceForm.Controls.Count - 1
If SourceForm.Controls .Name = cName Then cNum = A
Next A
' add some debug info if in debugmode
#If DebugMode = 1 Then
Debug.Print "Control Type=" + cType
Debug.Print "Control Name=" + cName
Debug.Print "Control Number=" + CStr(cNum)
#End If
' if we find control
If Not cNum = -1 Then
' Depending on type of control, what data we get
Select Case cType
Case "TextBox"
Line Input #FHandle, fData
fData = "": TXT = ""
Line Input #FHandle, fData
fData = "": BackColor = ""
While Not fData = "EndText"
If Not TXT = "" Then TXT = TXT + vbCrLf
TXT = TXT + fData
Line Input #FHandle, fData
Wend
' update control
SourceForm.Controls(cNum).Text = TXT
Case "CheckBox"
' we get the value
Line Input #FHandle, fData
' update control
SourceForm.Controls(cNum).Value = fData
Case "OptionButton"
' we get the value
Line Input #FHandle, fData
' update control
SourceForm.Controls(cNum).Value = fData
Case "ListBox"
' clear listbox
SourceForm.Controls(cNum).Clear
' get .text property
Line Input #FHandle, fData
SourceForm.Controls(cNum).Text = fData
' read past /startlist
Line Input #FHandle, fData
fData = "": TXT = ""
' Get List
While Not fData = "EndList"
If Not fData = "" Then SourceForm.Controls(cNum).AddItem fData
Line Input #FHandle, fData
Wend
' get listindex
Line Input #FHandle, fData
SourceForm.Controls(cNum).ListIndex = Val(fData)
Case "ComboBox"
' Clear combobox
SourceForm.Controls(cNum).Clear
' Get Text
Line Input #FHandle, fData
SourceForm.Controls(cNum).Text = fData
' readpast /startlist
Line Input #FHandle, fData
fData = "": TXT = ""
' get list
While Not fData = "EndList"
If Not fData = "" Then SourceForm.Controls(cNum).AddItem fData
Line Input #FHandle, fData
Wend
End Select ' what type of control
End If ' if we found control
' read till seperator
fData = ""
While Not fData = "|<->|"
Line Input #FHandle, fData
Wend
Wend ' not end of File (EOF)
' close file
#If DebugMode = 1 Then
Debug.Print "Closing file.."
Debug.Print "<------------------------------------------------------"
#End If
Close #FHandle
Exit Sub
fError: ' Simple error handler
C = MsgBox("Error in LoadFormState. " + Err.Description + ", Number=" + CStr(Err.Number), vbAbortRetryIgnore)
If C = vbIgnore Then Resume Next
If C = vbRetry Then Resume
' else abort
End Sub
What the code does is it saves all textboxes, listboxes ext. in a form. Then you can restore the saved properties.
What I would like is have it save the textboxes color too and reload it back. I'm not to good with coding of this sort.
Any help would be greatly appreciated.
* This is a module, you call it with Module1.SaveFormState Form1
RE: Help with Visual Basic code by Dempsey on 01-21-2005 at 11:32 PM
When you say the textboxes colour, do you mean the colour of the text? If so use code: SourceForm.Controls(cNum).ForeColor
RE: Help with Visual Basic code by DJeX on 01-23-2005 at 04:55 AM
No I mean the color of the text box background. Say if I used SourceForm.Controls(cNum).BackColor how would I reload that? Or would that work?
RE: Help with Visual Basic code by Mike on 01-23-2005 at 07:24 AM
This should work:
code: Option Compare Text
Option Explicit
#Const DebugMode = 1 ' whether or not to show debug information
'@===========================================================================
' LoadFormState:
' Loads the state of controls from file
'
' Currently Supports: TextBox, CheckBox, OptionButton, Listbox, ComboBox
'============================================================================
Public Sub LoadFormState(ByVal SourceForm As Form)
Dim A As Long ' general purpose
'Dim B As Long
Dim C As Long
Dim TXT As String ' general purpose
Dim fData As String ' used to hold File Data
' these are variables used for controls data
Dim cType As String ' Type of control
Dim cName As String ' Name of control
Dim cNum As Integer ' number of control
' vars for the file
Dim FileName As String ' where to save to
Dim FHandle As Long ' FileHandle
' error handling code
'On Error GoTo fError
' we create a filename based on the formname
FileName = App.Path & "\" & SourceForm.Name & ".set"
' abort if file does not exist
If LenB(Dir(FileName)) = 0 Then
#If DebugMode = 1 Then
Debug.Print "File Not found:" & FileName
#End If
Exit Sub
End If
' Get a filehandle
FHandle = FreeFile()
' open the file
#If DebugMode = 1 Then
Debug.Print "------------------------------------------------------>"
Debug.Print "Loading FormState:" & SourceForm.Name
Debug.Print "FileName:" & FileName
#End If
Open FileName For Input As FHandle
' go through file
Do While Not EOF(FHandle)
Line Input #FHandle, cType
Line Input #FHandle, cName
' Get control number
cNum = -1
For A = 0 To SourceForm.Controls.Count - 1
If SourceForm.Controls .Name = cName Then
cNum = A
End If
Next A
' add some debug info if in debugmode
#If DebugMode = 1 Then
Debug.Print "Control Type=" & cType
Debug.Print "Control Name=" & cName
Debug.Print "Control Number=" & CStr(cNum)
#End If
' if we find control
If Not cNum = -1 Then
' Depending on type of control, what data we get
Select Case cType
Case "TextBox"
Line Input #FHandle, fData
fData = ""
TXT = ""
Line Input #FHandle, fData
fData = ""
BackColor = ""
SourceForm.Controls(cNum).BackColor = fData
Do While Not fData = "EndText"
If Not LenB(TXT) = 0 Then
TXT = TXT + vbNewLine
End If
TXT = TXT + fData
Line Input #FHandle, fData
Loop
' update control
SourceForm.Controls(cNum).Text = TXT
Case "CheckBox"
' we get the value
Line Input #FHandle, fData
' update control
SourceForm.Controls(cNum).Value = fData
Case "OptionButton"
' we get the value
Line Input #FHandle, fData
' update control
SourceForm.Controls(cNum).Value = fData
Case "ListBox"
' clear listbox
SourceForm.Controls(cNum).Clear
' get .text property
Line Input #FHandle, fData
SourceForm.Controls(cNum).Text = fData
' read past /startlist
Line Input #FHandle, fData
fData = ""
TXT = ""
' Get List
Do While Not fData = "EndList"
If Not LenB(fData) = 0 Then
SourceForm.Controls(cNum).AddItem fData
End If
Line Input #FHandle, fData
Loop
' get listindex
Line Input #FHandle, fData
SourceForm.Controls(cNum).ListIndex = Val(fData)
Case "ComboBox"
' Clear combobox
SourceForm.Controls(cNum).Clear
' Get Text
Line Input #FHandle, fData
SourceForm.Controls(cNum).Text = fData
' readpast /startlist
Line Input #FHandle, fData
fData = ""
TXT = ""
' get list
Do While Not fData = "EndList"
If Not LenB(fData) = 0 Then
SourceForm.Controls(cNum).AddItem fData
End If
Line Input #FHandle, fData
Loop
End Select ' what type of control
End If ' if we found control
' read till seperator
fData = ""
Do While Not fData = "|<->|"
Line Input #FHandle, fData
Loop
Loop ' not end of File (EOF)
' close file
#If DebugMode = 1 Then
Debug.Print "Closing file.."
Debug.Print "<------------------------------------------------------"
#End If
Close #FHandle
Exit Sub
End Sub
'@===========================================================================
' SaveFormState:
' Saves the state of controls to a file
'
' Currently Supports: TextBox, CheckBox, OptionButton, Listbox, ComboBox
'=============================================================================
Public Sub SaveFormState(ByVal SourceForm As Form)
Dim A As Long ' general purpose
Dim B As Long
Dim C As Long
Dim FileName As String ' where to save to
Dim FHandle As Long ' FileHandle
' error handling code
On Error GoTo fError
' we create a filename based on the formname
FileName = App.Path & "\" & SourceForm.Name & ".set"
' Get a filehandle
FHandle = FreeFile()
' open the file
#If DebugMode = 1 Then
Debug.Print "--------------------------------------------------------->"
Debug.Print "Saving Form State:" & SourceForm.Name
Debug.Print "FileName=" & FileName
#End If
Open FileName For Output As FHandle
' loop through all controls
' first we save the type then the name
For A = 0 To SourceForm.Controls.Count - 1
#If DebugMode = 1 Then
Debug.Print "Saving control:" & SourceForm.Controls .Name
#End If
' if its textbox we save the .text property
If TypeOf SourceForm.Controls Is TextBox Then
Print #FHandle, "TextBox"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, "StartText"
Print #FHandle, SourceForm.Controls .Text
Print #FHandle, "EndText"
Print #FHandle, SourceForm.Controls .BackColor
' print a separator
Print #FHandle, "|<->|"
End If
' if its a checkbox we save the .value property
If TypeOf SourceForm.Controls Is CheckBox Then
Print #FHandle, "CheckBox"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, Str$(SourceForm.Controls .Value)
' print a separator
Print #FHandle, "|<->|"
End If
' if its a option button we save its value
If TypeOf SourceForm.Controls Is OptionButton Then
Print #FHandle, "OptionButton"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, Str$(SourceForm.Controls .Value)
' print a separator
Print #FHandle, "|<->|"
End If
' if its a listbox we save the .text and list contents
If TypeOf SourceForm.Controls Is ListBox Then
Print #FHandle, "ListBox"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, SourceForm.Controls .Text
Print #FHandle, "StartList"
For B = 0 To SourceForm.Controls .ListCount - 1
Print #FHandle, SourceForm.Controls .List
Next B
Print #FHandle, "EndList"
' save listindex
Print #FHandle, CStr(SourceForm.Controls .ListIndex)
' print a separator
Print #FHandle, "|<->|"
End If
' if its a combobox, save .text and list items
If TypeOf SourceForm.Controls Is ComboBox Then
Print #FHandle, "ComboBox"
Print #FHandle, SourceForm.Controls .Name
Print #FHandle, SourceForm.Controls .Text
Print #FHandle, "StartList"
For B = 0 To SourceForm.Controls .ListCount - 1
Print #FHandle, SourceForm.Controls .List
Next B
Print #FHandle, "EndList"
' print a separator
Print #FHandle, "|<->|"
End If
Next A
' close file
#If DebugMode = 1 Then
Debug.Print "Closing File."
Debug.Print "<----------------------------------------------------------"
#End If
Close #FHandle
' stop error handler
On Error GoTo 0
Exit Sub
fError:
' Simple error handler
C = MsgBox("Error in SaveFormState. " & Err.Description & ", Number=" & CStr(Err.Number), vbAbortRetryIgnore)
If C = vbIgnore Then
Resume Next
End If
If C = vbRetry Then
Resume
End If
' else abort
End Sub
Haven't tested it though...
RE: Help with Visual Basic code by DJeX on 01-23-2005 at 08:50 PM
Mike2, it saves the colors fine but I get a Type Missmatch when I go to load them back in. It highlights this line.
SourceForm.Controls(cNum).BackColor = fData
Any ideas on how to fix this?
Oh BTW you have to put Dim BackColor As String at the top of the code or else you get a Varible not defined error.
RE: Help with Visual Basic code by Mike on 01-23-2005 at 08:54 PM
Try then:
SourceForm.Controls(cNum).BackColor = CLong(fData)
RE: Help with Visual Basic code by DJeX on 01-23-2005 at 09:00 PM
It says Sub or Funtion not defined and it highlights CLong
So then if I put Dim CLong As Integer it says Expected array. Same with Dim CLong As String.
RE: Help with Visual Basic code by Mike on 01-23-2005 at 09:03 PM
Bah, sorry, it is CLng ...
So:
SourceForm.Controls(cNum).BackColor = CLng(fData)
RE: Help with Visual Basic code by DJeX on 01-23-2005 at 10:58 PM
Humm now it says Type mismatch and highlights
SourceForm.Controls(cNum).BackColor = CLng(fData)
RE: Help with Visual Basic code by Mike on 01-24-2005 at 11:52 AM
Bah...
Then try replacing the LoadFormState sub with:
code: Public Sub LoadFormState(ByVal SourceForm As Form)
Dim A As Long ' general purpose
'Dim B As Long
Dim C As Long
Dim TXT As String ' general purpose
Dim fData As String ' used to hold File Data
' these are variables used for controls data
Dim cType As String ' Type of control
Dim cName As String ' Name of control
Dim cNum As Integer ' number of control
' vars for the file
Dim FileName As String ' where to save to
Dim FHandle As Long ' FileHandle
' error handling code
'On Error GoTo fError
' we create a filename based on the formname
FileName = App.Path & "\" & SourceForm.Name & ".set"
' abort if file does not exist
If LenB(Dir(FileName)) = 0 Then
#If DebugMode = 1 Then
Debug.Print "File Not found:" & FileName
#End If
Exit Sub
End If
' Get a filehandle
FHandle = FreeFile()
' open the file
#If DebugMode = 1 Then
Debug.Print "------------------------------------------------------>"
Debug.Print "Loading FormState:" & SourceForm.Name
Debug.Print "FileName:" & FileName
#End If
Open FileName For Input As FHandle
' go through file
Do While Not EOF(FHandle)
Line Input #FHandle, cType
Line Input #FHandle, cName
' Get control number
cNum = -1
For A = 0 To SourceForm.Controls.Count - 1
If SourceForm.Controls .Name = cName Then
cNum = A
End If
Next A
' add some debug info if in debugmode
#If DebugMode = 1 Then
Debug.Print "Control Type=" & cType
Debug.Print "Control Name=" & cName
Debug.Print "Control Number=" & CStr(cNum)
#End If
' if we find control
If Not cNum = -1 Then
' Depending on type of control, what data we get
Select Case cType
Case "TextBox"
Line Input #FHandle, fData
fData = ""
TXT = ""
Line Input #FHandle, fData
Debug.Print fData
SourceForm.Controls(cNum).BackColor =CLng(fData)
fData = ""
BackColor = ""
Do While Not fData = "EndText"
If Not LenB(TXT) = 0 Then
TXT = TXT + vbNewLine
End If
TXT = TXT + fData
Line Input #FHandle, fData
Loop
' update control
SourceForm.Controls(cNum).Text = TXT
Case "CheckBox"
' we get the value
Line Input #FHandle, fData
' update control
SourceForm.Controls(cNum).Value = fData
Case "OptionButton"
' we get the value
Line Input #FHandle, fData
' update control
SourceForm.Controls(cNum).Value = fData
Case "ListBox"
' clear listbox
SourceForm.Controls(cNum).Clear
' get .text property
Line Input #FHandle, fData
SourceForm.Controls(cNum).Text = fData
' read past /startlist
Line Input #FHandle, fData
fData = ""
TXT = ""
' Get List
Do While Not fData = "EndList"
If Not LenB(fData) = 0 Then
SourceForm.Controls(cNum).AddItem fData
End If
Line Input #FHandle, fData
Loop
' get listindex
Line Input #FHandle, fData
SourceForm.Controls(cNum).ListIndex = Val(fData)
Case "ComboBox"
' Clear combobox
SourceForm.Controls(cNum).Clear
' Get Text
Line Input #FHandle, fData
SourceForm.Controls(cNum).Text = fData
' readpast /startlist
Line Input #FHandle, fData
fData = ""
TXT = ""
' get list
Do While Not fData = "EndList"
If Not LenB(fData) = 0 Then
SourceForm.Controls(cNum).AddItem fData
End If
Line Input #FHandle, fData
Loop
End Select ' what type of control
End If ' if we found control
' read till seperator
fData = ""
Do While Not fData = "|<->|"
Line Input #FHandle, fData
Loop
Loop ' not end of File (EOF)
' close file
#If DebugMode = 1 Then
Debug.Print "Closing file.."
Debug.Print "<------------------------------------------------------"
#End If
Close #FHandle
Exit Sub
End Sub
RE: Help with Visual Basic code by DJeX on 01-24-2005 at 08:30 PM
Ermmm did you try this code? because you forgot to Dim BackColor As String. And it still has the same error, Type Missmatch and it highlights SourceForm.Controls(cNum).BackColor = CLng(fData)
|