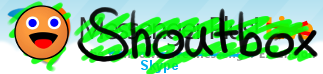
PHP Help, file upload - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Skype & Technology (/forumdisplay.php?fid=9)
+---- Forum: Tech Talk (/forumdisplay.php?fid=17)
+----- Thread: PHP Help, file upload (/showthread.php?tid=37731)
PHP Help, file upload by matty on 01-30-2005 at 10:14 PM
Hey everyone, I have been trying to write a File Upload script for a site I am doing but I can't seem to find a working script.
If anyone could help me out that would be great. I have even tried ones on PSCode but they don't work. Here is the infor for the server that it will be on.
RE: PHP Help, file upload by KeyStorm on 01-30-2005 at 10:22 PM
you just need a <input type="file"/ name="myFile"> input field and then an autoglobal array $_FILES['myFile'] will be available. This array has a second dimension with following keys:
name - the name of the uploaded file with extension
type - the filetype (mime type)
size - amount of bytes of the file
tmp_name - absolute path to the temporary file (its name is random)
The rest (storing to a folder, uploading to a database, showing the file) goes to your imagination. 
If you need some more help, just tell us.
RE: PHP Help, file upload by L. Coyote on 01-30-2005 at 10:58 PM
An example of a correct form:
<form enctype="multipart/form-data" action="_URL_" method="post">
It's very important that you put that bolded part. Else, it won't work.
More info here.
RE: PHP Help, file upload by segosa on 01-31-2005 at 06:28 AM
I made a file upload script the other day, it's about 100 lines. If you want me to send you it contact me on MSN Messenger, Matty.
RE: PHP Help, file upload by megamuff on 02-01-2005 at 04:02 PM
code: <?php
if ($HTTP_POST_VARS['submit']) {
print_r($HTTP_POST_FILES);
if (!is_uploaded_file($HTTP_POST_FILES['file']['tmp_name'])) {
$error = "You did not upload a file!";
unlink($HTTP_POST_FILES['file']['tmp_name']);
// assign error message, remove uploaded file, redisplay form.
} else {
//a file was uploaded
$maxfilesize=125829120;
if ($HTTP_POST_FILES['file']['size'] > $maxfilesize) {
$error = "file is too large";
unlink($HTTP_POST_FILES['file']['tmp_name']);
// assign error message, remove uploaded file, redisplay form.
} else {
if ($HTTP_POST_FILES['file']['type'] != "image/gif" AND $HTTP_POST_FILES['file']['type'] != "image/pjpeg" AND $HTTP_POST_FILES['file']['type'] != "image/bmp" AND $HTTP_POST_FILES['file']['type'] != "video/mpeg" AND $HTTP_POST_FILES['file']['type'] != "video/quicktime" AND $HTTP_POST_FILES['file']['type'] != "video/x-msvideo" AND $HTTP_POST_FILES['file']['type'] != "image/png" AND $HTTP_POST_FILES['file']['type'] != "application/x-shockwave-flash") {
$error = "This file type is not allowed";
unlink($HTTP_POST_FILES['file']['tmp_name']);
// assign error message, remove uploaded file, redisplay form.
} else {
//File has passed all validation, copy it to the final destination and remove the temporary file:
copy($HTTP_POST_FILES['file']['tmp_name'],"up/".$HTTP_POST_FILES['file']['name']);
unlink($HTTP_POST_FILES['file']['tmp_name']);
print "File has been successfully uploaded!";
exit;
}
}
}
}
?>
<html>
<head>
<title>File Uploader</title>
</head>
<center>
<form action="<?=$PHP_SELF?>" method="post" enctype="multipart/form-data">
<?=$error?>
<br>if a file name has spaces in it, rename the file and take out the spaces before you upload it!!<br>
Choose a file to upload br>
<input type="file" name="file"><br>
<input type="submit" name="submit" value="submit">
</form>
</center>
</body>
</html>
RE: PHP Help, file upload by segosa on 02-01-2005 at 09:44 PM
He already got the code, I sent him my script 
code: if ($HTTP_POST_FILES['file']['type'] != "image/gif" AND $HTTP_POST_FILES['file']['type'] != "image/pjpeg" AND $HTTP_POST_FILES['file']['type'] != "image/bmp" AND $HTTP_POST_FILES['file']['type'] != "video/mpeg" AND $HTTP_POST_FILES['file']['type'] != "video/quicktime" AND $HTTP_POST_FILES['file']['type'] != "video/x-msvideo" AND $HTTP_POST_FILES['file']['type'] != "image/png" AND $HTTP_POST_FILES['file']['type'] != "application/x-shockwave-flash") {
Talk about inefficient
RE: PHP Help, file upload by megamuff on 02-02-2005 at 03:03 AM
quote: Originally posted by Segosa
He already got the code, I sent him my script 
code: if ($HTTP_POST_FILES['file']['type'] != "image/gif" AND $HTTP_POST_FILES['file']['type'] != "image/pjpeg" AND $HTTP_POST_FILES['file']['type'] != "image/bmp" AND $HTTP_POST_FILES['file']['type'] != "video/mpeg" AND $HTTP_POST_FILES['file']['type'] != "video/quicktime" AND $HTTP_POST_FILES['file']['type'] != "video/x-msvideo" AND $HTTP_POST_FILES['file']['type'] != "image/png" AND $HTTP_POST_FILES['file']['type'] != "application/x-shockwave-flash") {
Talk about inefficient 
got a better way to allow only those file types through?
RE: PHP Help, file upload by WDZ on 02-02-2005 at 03:55 AM
I'd probably do something like this...
code: $types = array("image/gif", "image/pjpeg", "image/bmp", "video/mpeg", "video/x-msvideo", "image/png", "application/x-shockwave-flash");
if(in_array($HTTP_POST_FILES['file']['type'], $types)) {
RE: PHP Help, file upload by L. Coyote on 02-02-2005 at 04:06 AM
quote: Originally posted by WDZ
I'd probably do something like this...
code: $types = array("image/gif", "image/pjpeg", "image/bmp", "video/mpeg", "video/x-msvideo", "image/png", "application/x-shockwave-flash");
if(in_array($HTTP_POST_FILES['file']['type'], $types)) {

Isn't it better to use $_FILES?
RE: PHP Help, file upload by WDZ on 02-02-2005 at 04:09 AM
quote: Originally posted by Leo
Isn't it better to use $_FILES? 
Yeah, unless your version of PHP is ancient. I didn't use it because the original code didn't use it.
RE: PHP Help, file upload by segosa on 02-02-2005 at 06:30 AM
Can I just add a question while we're on the subject of uploading:
The mime-type is sent by the browser in the headers before the file is sent, or is it stored inside the file somehow? The reason I ask this is that if it's sent by the browser it is possible to fake it, and so upload a .php file pretending to be image/png or something else because only the mime-types are checked...
RE: PHP Help, file upload by megamuff on 02-02-2005 at 06:41 AM
quote: Originally posted by Segosa
Can I just add a question while we're on the subject of uploading:
The mime-type is sent by the browser in the headers before the file is sent, or is it stored inside the file somehow? The reason I ask this is that if it's sent by the browser it is possible to fake it, and so upload a .php file pretending to be image/png or something else because only the mime-types are checked...
in order to upload the .php file as an image as you are describing, a setting in apache would need to be changed in the mime.types file.
quote: Originally posted by WDZ
I'd probably do something like this...
code: $types = array("image/gif", "image/pjpeg", "image/bmp", "video/mpeg", "video/x-msvideo", "image/png", "application/x-shockwave-flash");
if(in_array($HTTP_POST_FILES['file']['type'], $types)) {

thanks.
RE: PHP Help, file upload by WDZ on 02-02-2005 at 07:05 AM
It is completely possible to fake a mime type when uploading a file, so I strongly recommend also checking the extension, especially if you're going to store the file on your web server in a publically-accessable location with its original filename.
I assume that browsers get their mime types from the registry. For example, if you browse to HKEY_CLASSES_ROOT\.jpg, you will see a value called "Content Type" set to "image/jpeg." If a certain type of file is not in the registry, a generic type like "application/octet-stream" or "text/plain" will be used.
Another possibility is that the browser reads the first few bytes of the file, looking for common headers, such as "GIF89a" for a gif image. I think I'll research this further... 
quote: Originally posted by megamuff
in order to upload the .php file as an image as you are describing, a setting in apache would need to be changed in the mime.types file.
Uhh, you're obviously not on the same page.
RE: PHP Help, file upload by KeyStorm on 02-02-2005 at 11:14 AM
Maybe a server-side mimetyping script or class wouldn't be any bad... 
Anyway, I gave Matty some hints on Messenger already. He only needs to assure he's uploading mp3's, so extension and mime/type check is the least he can do for now.
RE: RE: PHP Help, file upload by segosa on 02-02-2005 at 03:39 PM
quote: Originally posted by WDZ
It is completely possible to fake a mime type when uploading a file, so I strongly recommend also checking the extension, especially if you're going to store the file on your web server in a publically-accessable location with its original filename.
I assume that browsers get their mime types from the registry. For example, if you browse to HKEY_CLASSES_ROOT\.jpg, you will see a value called "Content Type" set to "image/jpeg." If a certain type of file is not in the registry, a generic type like "application/octet-stream" or "text/plain" will be used.
Another possibility is that the browser reads the first few bytes of the file, looking for common headers, such as "GIF89a" for a gif image. I think I'll research this further... 
Well, I tried it out.
I found out where Firefox got its mime-types from (C:\Documents and Settings\<username>\Application Data\Mozilla\Firefox\Profiles\<profile>\mimeTypes.rdf) and added an entry for .php:
code:
<RDF: Description RDF:about="urn:mimetype:image/png"
NC:value="image/png"
NC:editable="true"
NC:fileExtensions="php"
NC: description="PHP File">
<NC:handlerProp RDF:resource="urn:mimetype:handler:image/png"/>
</RDF: Description>
making Firefox think it's actually image/png. I created a PHP upload script which only made a check on the mime-type:
code: if ($_FILES['file']['type'] == "image/png")
and tried uploading a .png, it worked fine. I created a .php and uploaded, and that uploaded fine too.
RE: PHP Help, file upload by WDZ on 02-02-2005 at 04:02 PM
quote: Originally posted by Segosa
I found out where Firefox got its mime-types from (C:\Documents and Settings\<username>\Application Data\Mozilla\Firefox\Profiles\<profile>\mimeTypes.rdf) and added an entry for .php:
Ah, interesting. Maybe only MSIE gets its types from the registry. I wonder about Opera now... 
Hmm... I found a section in the config file (opera6.ini) where many mime types are associated with extensions, so that could be it.
|