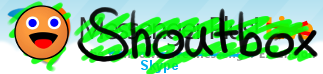
[C#] Write to MsgPlus Event Log - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Messenger Plus! for Live Messenger (/forumdisplay.php?fid=4)
+---- Forum: Scripting (/forumdisplay.php?fid=39)
+----- Forum: Plug-Ins (/forumdisplay.php?fid=28)
+------ Thread: [C#] Write to MsgPlus Event Log (/showthread.php?tid=51333)
[C#] Write to MsgPlus Event Log by andersona91 on 10-03-2005 at 06:00 PM
Hello folks,
Just a quick question, how do you write to the MsgPlus event log in C#?
Thanks!
---- Anderson ----
RE: [C#] Write to MsgPlus Event Log by J-Thread on 10-03-2005 at 06:21 PM
Editted again (this one does work):
code: const int HWND_BROADCAST = 0xFFFF;
[DllImport("user32", EntryPoint="RegisterWindowMessageA")] public static extern int RegisterWindowMessage([MarshalAs(UnmanagedType.LPStr)]string lpString);
[DllImport("user32", EntryPoint="SendMessageA")] public static extern int SendMessage(int hwnd, int wMsg, int wParam, int lParam);
private struct MPL_EVENTLOG_INFO
{
public int sUser;
public int sEvent;
public int nReserved;
}
public bool AddEventEntry(string sUser, string sEvent)
{
MPL_EVENTLOG_INFO info = new MPL_EVENTLOG_INFO();
info.nReserved = 0;
System.Runtime.InteropServices.GCHandle userPtr = new System.Runtime.InteropServices.GCHandle();
if(sUser.Length < 1)
{
info.sUser = 0;
}
else
{
userPtr = System.Runtime.InteropServices.GCHandle.Alloc(sUser, System.Runtime.InteropServices.GCHandleType.Pinned);
info.sUser = userPtr.AddrOfPinnedObject().ToInt32();
}
System.Runtime.InteropServices.GCHandle eventPtr = new System.Runtime.InteropServices.GCHandle();
if(sEvent.Length < 1)
{
info.sEvent = 0;
}
else
{
eventPtr = System.Runtime.InteropServices.GCHandle.Alloc(sEvent, System.Runtime.InteropServices.GCHandleType.Pinned);
info.sEvent = eventPtr.AddrOfPinnedObject().ToInt32();
}
int nMsg = RegisterWindowMessage("MessengerPlus_AddEventLog");
System.Runtime.InteropServices.GCHandle infoPtr = System.Runtime.InteropServices.GCHandle.Alloc(info, System.Runtime.InteropServices.GCHandleType.Pinned);
int nResult = SendMessage(HWND_BROADCAST, nMsg, infoPtr.AddrOfPinnedObject().ToInt32(), 1);
if(info.sEvent != 0)
{
eventPtr.Free();
}
if(info.sUser != 0)
{
userPtr.Free();
}
infoPtr.Free();
return true;
}
RE: [C#] Write to MsgPlus Event Log by J-Thread on 10-04-2005 at 07:14 PM
Did you test it already? Does it work?
RE: [C#] Write to MsgPlus Event Log by Jedimark on 10-04-2005 at 09:50 PM
J: I wrote very similar code to you when I was trying to integrate my Music Logger Plus plugin with the MsgPlus event log but couldnt for the life get it to actually work (there were no compile problems). I tried your code aswell and no luck either.
I'm guessing that the call is getting rejected by MsgPlus for some security reasons.
How should I invoke the method?
andersona91: Did you get it to work?
- Mark
RE: [C#] Write to MsgPlus Event Log by J-Thread on 10-05-2005 at 05:48 AM
Ok I'll test it myself later. I assume that patchou did test his VB.NET code, so that must be correct.
If the VB.NET code works, this should also work in my opinion... Sorry I made a little mistake in my code!
It does work now . Make sure you've got the option to log things on . By the way, it isn't possible to write to the conversation logs, only to the "actions log" (don't know how it's called, in dutch it's the "gebeurtenissenlogboek".
RE: [C#] Write to MsgPlus Event Log by CookieRevised on 10-05-2005 at 02:35 PM
quote: Originally posted by J-Thread
(don't know how it's called, in dutch it's the "gebeurtenissenlogboek").
Event Log
----
ps: try to not double post... If you wanna add something, edit your previous post...
RE: [C#] Write to MsgPlus Event Log by Jedimark on 10-06-2005 at 07:12 PM
J-Thread: Your code works (sort of) and mine doesn't so I'll just use yours lol 
Anyway, I have the AddEventEntry in a class called Utilities.cs and I made it "public static bool" so I could then do:
code: MLP.Utilities.AddEventEntry(thisContact, language[18] + " " + thisTitle + " " + language[19] + " " + thisArtist);
But it doesn't work.
However, I can write to the EventLog if I call the method from "MainForm_Load", why is this?
The method I want it to be called from is simply:
code: private bool addEventLog(string thisContact, string thisTitle, string thisArtist)
{
RegistryKey rootPath = Registry.CurrentUser.CreateSubKey("Software\\Jedimark\\Music Logger Plus");
string logStatus = rootPath.GetValue("LogToPlus").ToString();
if (logStatus.Equals("true"))
{
MLP.Utilities.AddEventEntry(thisContact, language[18] + " " + thisTitle + " " + language[19] + " " + thisArtist);
return true;
}
return false;
}
(and yes, the if statement does return "true" so it is executing the call)
RE: [C#] Write to MsgPlus Event Log by J-Thread on 10-06-2005 at 08:17 PM
About that static method, it should work indeed. I don't know what the problem is...
About the code you posted, I see a huge bug!
code: private bool addEventLog(string thisContact, string thisTitle, string thisArtist)
{
RegistryKey rootPath = Registry.CurrentUser.OpenSubKey("Software\\Jedimark\\Music Logger Plus");
string logStatus = rootPath.GetValue("LogToPlus").ToString();
if (logStatus.Equals("true"))
{
MLP.Utilities.AddEventEntry(thisContact, language[18] + " " + thisTitle + " " + language[19] + " " + thisArtist);
return true;
}
return false;
}
I hope you use OpenSubKey and not CreateSubkey!
There is a better way to store booleans in the registry btw. Use a DWORD value, set it 1 for true and 0 for false.
Does it work when you make your addEventLog function static also?
RE: [C#] Write to MsgPlus Event Log by Jedimark on 10-06-2005 at 08:48 PM
Yeah you're right it should be OpenSubKey, a rushed method!
The addEventLog doesn't work if it's static, I tried that already! I'm actually well and truely stuck with this one... this is what working full time has done to me
RE: [C#] Write to MsgPlus Event Log by J-Thread on 10-07-2005 at 08:08 AM
Ok I did test it and this works:
In Utilities.cs, make the following code:
code: [ComVisible(false)]
public class Utilities
{
const int HWND_BROADCAST = 0xFFFF;
[DllImport("user32", EntryPoint="RegisterWindowMessageA")] public static extern int RegisterWindowMessage([MarshalAs(UnmanagedType.LPStr)]string lpString);
[DllImport("user32", EntryPoint="SendMessageA")] public static extern int SendMessage(int hwnd, int wMsg, int wParam, int lParam);
public Utilities()
{
}
public static bool AddEventEntry(string sUser, string sEvent)
....(here the rest of my function)
Then your other function should be:
code: private bool addEventLog(string thisContact, string thisTitle, string thisArtist)
{
RegistryKey rootPath = Registry.CurrentUser.OpenSubKey("Software\\Jedimark\\Music Logger Plus");
string logStatus = rootPath.GetValue("LogToPlus").ToString();
if (logStatus.Equals("true"))
{
Utilities.AddEventEntry(thisContact, language[18] + " " + thisTitle + " " + language[19] + " " + thisArtist);
return true;
}
return false;
}
So remove the namespace. This should all work. By the way, do you see an error or something? How do you know it isn't working?
RE: [C#] Write to MsgPlus Event Log by Jedimark on 10-07-2005 at 04:03 PM
Still wont work, I'm sure there's a really simple thing wrong with the code somewhere! Frustrating huh!
It does work in the MainForm_Load method though 
Wanna chat about this on MSN? My address is my username at gmail dot com.
- Jedimark
RE: [C#] Write to MsgPlus Event Log by J-Thread on 10-07-2005 at 06:30 PM
Ok I don't have time now, add you later
|