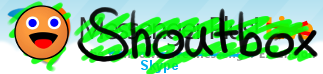
C# Programming Help - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Skype & Technology (/forumdisplay.php?fid=9)
+---- Forum: Tech Talk (/forumdisplay.php?fid=17)
+----- Thread: C# Programming Help (/showthread.php?tid=52935)
C# Programming Help by matty on 11-14-2005 at 12:31 AM
My friend is having trouble with an assignment and I don't know enough of C# to help her.
code: using System;
namespace DateChecker
{
class Class1
{
[STAThread]
static void Main(string[] args)
{
class Introduction
{
static void Intro()
{
Console.WriteLine("Welcome to the DateChecker Program!");
Console.WriteLine("***********************************");
}
class Input_Day
{
static void ValidDay(out string InputDay)
{
InputDay = "Please enter the day of the month (DD): ";
int sUserDay = Int32.Parse(Console.ReadLine());
}
class Input_Month
{
static void ValidMonth(out string InputMonth)
{
InputMonth = "Please enter the month (MM): ";
int sUserMonth = Int32.Parse(Console.ReadLine());
}
class Input_Year
{
static void ValidYear(out string InputYear)
{
InputYear = "Please enter the year (YYYY): ";
int sUserYear = Int32.Parse(Console.ReadLine());
}
}
switch(Input_Month)
{
case 0:
case 2:
case 4:
case 6:
case 7:
case 9:
case 11:
sUserDay = case 0,2,4,6,7,9,11 <= 31;
//If it has 31 days in the month
break;
case 3:
case 5:
case 8:
case 10:
sUserDay = case 3,5,8,10 <= 30;
//If it has 30 days in the month
break;
default:
sUserDay = default <= 28;
break;
}
}
}
}
}
}
Here are the errors she gets
![[Image: attachment.php?pid=564773]](http://shoutbox.menthix.net/attachment.php?pid=564773)
The program is supposed to allow the user to enter day then month then year (numerically) and the program is to decide if it is a valid date taking into consideration leap years.
Any help is appreciated.
RE: C# Programming Help by matty on 11-14-2005 at 01:54 AM
bump
Here is a text file of the code so its easier to read.
RE: C# Programming Help by ^_^ on 11-14-2005 at 02:02 AM
Remember that you need to close all the { that you open, try to change the = thing for a == ... what program are you using? i use Dev c++ 4.9 and im quite good at it
RE: C# Programming Help by matty on 11-14-2005 at 02:05 AM
quote: Originally posted by ^_^
Remember that you need to close all the { that you open, try to change the = thing for a == ... what program are you using? i use Dev c++ 4.9 and im quite good at it
Each } is closed properly and == doesn't work. I tried working on it in VisualStudio.Net (Which is what she uses).
RE: C# Programming Help by brian on 11-14-2005 at 02:37 AM
Missing } at end?
RE: C# Programming Help by matty on 11-14-2005 at 02:42 AM
quote: Originally posted by nvez
Missing } at end?
No for some reason if you remove the code including class introduction then its fine with no errors.
RE: C# Programming Help by brian on 11-14-2005 at 02:57 AM
It's something with the namespace part..
Try: namespace DateChecker;
she? 
* brian runs
RE: C# Programming Help by matty on 11-14-2005 at 03:08 AM
quote: Originally posted by nvez
It's something with the namespace part..
Try: namespace DateChecker;
she? 
* nvez runs
Nope produces more errors.
RE: C# Programming Help by brian on 11-14-2005 at 03:10 AM
What about removing the { .. } after namespace DateChecker ?
Mehish, tbh C++ is easier for such stuff.
RE: C# Programming Help by matty on 11-14-2005 at 03:29 AM
quote: Originally posted by nvez
What about removing the { .. } after namespace DateChecker ?
Mehish, tbh C++ is easier for such stuff.
Nope still recieving errors. Saying its missing {
RE: C# Programming Help by ShawnZ on 11-14-2005 at 03:31 AM
quote: Originally posted by Matty
quote: Originally posted by nvez
What about removing the { .. } after namespace DateChecker ?
Mehish, tbh C++ is easier for such stuff.
Nope still recieving errors. Saying its missing {
Maybe its because you're missing a { !!
RE: C# Programming Help by matty on 11-14-2005 at 03:34 AM
quote: Originally posted by ShawnZ
quote: Originally posted by Matty
quote: Originally posted by nvez
What about removing the { .. } after namespace DateChecker ?
Mehish, tbh C++ is easier for such stuff.
Nope still recieving errors. Saying its missing {
Maybe its because you're missing a { !!
I have gone over it. All opens have closes.
RE: C# Programming Help by ShawnZ on 11-14-2005 at 03:34 AM
quote: Originally posted by Matty
quote: Originally posted by ShawnZ
quote: Originally posted by Matty
quote: Originally posted by nvez
What about removing the { .. } after namespace DateChecker ?
Mehish, tbh C++ is easier for such stuff.
Nope still recieving errors. Saying its missing {
Maybe its because you're missing a { !!
I have gone over it. All opens have closes.
So have I. One is left open.
Edit: and in case you still can't see it, http://shoutbox.menthix.net/attachment.php?pid=564838
In the words of optimism_, "keep your day job."
RE: RE: C# Programming Help by matty on 11-14-2005 at 04:26 AM
quote: Originally posted by ShawnZquote: Originally posted by Matty
Nope still recieving errors. Saying its missing {
Maybe its because you're missing a { !!
Well I fixed it and here is what I get for my troubles
![[Image: attachment.php?pid=564847]](http://shoutbox.menthix.net/attachment.php?pid=564847)
quote: Originally posted by ShawnZ
In the words of optimism_, "keep your day job."
I code in VB not C# and when I was editing it there were equal number of { as } but posted the original.
RE: C# Programming Help by ShawnZ on 11-14-2005 at 04:33 AM
Does [STAThread] need {...}?
quote: Originally posted by Matty
I code in VB not C# and when I was editing it there were equal number of { as } but posted the original.
BS, why are you getting a different error now then.
RE: C# Programming Help by Weyzza on 11-14-2005 at 04:34 AM
quote: Originally posted by ShawnZ
Does [STAThread] need {...}?
I don't think it does.
quote: Originally posted by http://www.csharp-station.com/Tutorials/Lesson16.aspx
Listing 16-1 also contains another attribute you're likely to see, the STAThreadAttribute attribute. You'll often see this attribute applied to the Main() method, indicating that this C# program should communicate with unmanaged COM code using the Single Threading Apartment . It is generally safe to use this attribute all the time because you never know when a 3rd party library you're using is going to be communicating with COM. The following excerpt shows how to use the STAThreadAttribute attribute:
[STAThread]
static void Main(string[] args)
...
RE: C# Programming Help by matty on 11-14-2005 at 04:46 AM
quote: Originally posted by ShawnZ
Does [STAThread] need {...}?
quote: Originally posted by Matty
I code in VB not C# and when I was editing it there were equal number of { as } but posted the original.
BS, why are you getting a different error now then.
Because the original code posted was from my friend unedited from me. After I posted saying they are all there is when I had edited myself.
Look if all your going to do is try and bash me then don't even bother posting.
|