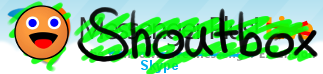
How to make a struct? - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Messenger Plus! for Live Messenger (/forumdisplay.php?fid=4)
+---- Forum: Scripting (/forumdisplay.php?fid=39)
+----- Thread: How to make a struct? (/showthread.php?tid=64296)
How to make a struct? by -=blu3+3y3s=- on 07-31-2006 at 08:51 PM
Hi, all!
I need to make Point and Rect structs for using GetWindowRect and GetCursorPos of user32.dll.
I try this:
code: struct Point
{
long x;
long y;
}
but when I apply, MsgPlus says me "Couldn't start script".
RE: How to make a struct? by Ezra on 07-31-2006 at 08:54 PM
If I understand what you mean this is want you want 
function Point()
{
this.x;
this.y;
}
mypointobj = new Point();
RE: How to make a struct? by -=blu3+3y3s=- on 07-31-2006 at 09:19 PM
I tried what you said but WLM bugges now when I launch the script.
I do this:
code: Interop.Call("user32.dll", "GetCursorPos", mypointobj);
MsgPlus.DisplayToast("Result", mypointobj.x + " - " + mypointobj.y);
How I can do to display the result?
RE: How to make a struct? by Ezra on 07-31-2006 at 09:25 PM
Hmm, I guess you'll have to do that another way.
Here is some code that I think would help you
Color Picker Made By Matty:
code: //Show color picker common dialog (Made By Matty);
//Create our CHOOSECOLOR data block
var CHOOSECOLOR = Interop.Allocate(36);
CHOOSECOLOR.WriteDWORD(0, 36); //DWORD lStructSize
CHOOSECOLOR.WriteDWORD(4, 0); //HWND hwndOwner
CHOOSECOLOR.WriteDWORD(8, 0); //HWND hInstance
CHOOSECOLOR.WriteDWORD(12, 0x000000FF); //COLORREF rgbResult (COLORREF = 0x00bbggrr)
var CustColors = Interop.Allocate(64); //Create an array of 16 COLORREFs for CustColors
CHOOSECOLOR.WriteDWORD(16, CustColors.DataPtr); //COLORREF *lpCustColors (pointer to our array)
CHOOSECOLOR.WriteDWORD(20, 3); //DWORD Flags (3 = 2 (CC_FULLOPEN) + 1 (CC_RGBINIT) )
CHOOSECOLOR.WriteDWORD(24, 0); //LPARAM lCustData
CHOOSECOLOR.WriteDWORD(28, 0); //LPCCHOOKPROC lpfnHook
CHOOSECOLOR.WriteDWORD(32, 0); //LPCTSTR lpTemplateName
//Open the dialog box
var result = Interop.Call('comdlg32.dll', 'ChooseColorA', CHOOSECOLOR);
//If the user pressed ok convert it to hex
if(result == 1){
//Get decimal values
var r = CHOOSECOLOR.ReadDWORD(12) & 0xFF;
var g = (CHOOSECOLOR.ReadDWORD(12) / 0x100) & 0xFF;
var b = (CHOOSECOLOR.ReadDWORD(12) / 0x10000) & 0xFF;
Debug.Trace('RGB: ' + r + ',' + g + ',' + b)
//Get hex values
var hexchars="0123456789ABCDEF";
var r = hexchars.charAt((r >> 4) & 0xf) + hexchars.charAt(r & 0xF);
var g = hexchars.charAt((g >> 4) & 0xf) + hexchars.charAt(g & 0xF);
var b = hexchars.charAt((b >> 4) & 0xf) + hexchars.charAt(b & 0xF);
Debug.Trace('HEX: ' + r + g + b);
}
RE: How to make a struct? by -dt- on 08-01-2006 at 12:00 AM
right well you people dont know what you're talking about you have to recreate the structure in memory and read it from memory, here you dont need to create the structure since it will be passed into point for you by GetCursorPos
code:
var point = Interop.Allocate(8);
Interop.Call("user32.dll", "GetCursorPos", point);
Debug.Trace("x: " + point.ReadDWORD(0));
Debug.Trace("y: " + point.ReadDWORD(4));
RE: How to make a struct? by Eljay on 08-01-2006 at 07:30 AM
quote: Originally posted by Ezra
Hmm, I guess you'll have to do that another way.
Here is some code that I think would help you
Color Picker Made By Matty:
code: //Show color picker common dialog (Made By Matty);
//Create our CHOOSECOLOR data block
var CHOOSECOLOR = Interop.Allocate(36);
CHOOSECOLOR.WriteDWORD(0, 36); //DWORD lStructSize
CHOOSECOLOR.WriteDWORD(4, 0); //HWND hwndOwner
CHOOSECOLOR.WriteDWORD(8, 0); //HWND hInstance
CHOOSECOLOR.WriteDWORD(12, 0x000000FF); //COLORREF rgbResult (COLORREF = 0x00bbggrr)
var CustColors = Interop.Allocate(64); //Create an array of 16 COLORREFs for CustColors
CHOOSECOLOR.WriteDWORD(16, CustColors.DataPtr); //COLORREF *lpCustColors (pointer to our array)
CHOOSECOLOR.WriteDWORD(20, 3); //DWORD Flags (3 = 2 (CC_FULLOPEN) + 1 (CC_RGBINIT) )
CHOOSECOLOR.WriteDWORD(24, 0); //LPARAM lCustData
CHOOSECOLOR.WriteDWORD(28, 0); //LPCCHOOKPROC lpfnHook
CHOOSECOLOR.WriteDWORD(32, 0); //LPCTSTR lpTemplateName
//Open the dialog box
var result = Interop.Call('comdlg32.dll', 'ChooseColorA', CHOOSECOLOR);
//If the user pressed ok convert it to hex
if(result == 1){
//Get decimal values
var r = CHOOSECOLOR.ReadDWORD(12) & 0xFF;
var g = (CHOOSECOLOR.ReadDWORD(12) / 0x100) & 0xFF;
var b = (CHOOSECOLOR.ReadDWORD(12) / 0x10000) & 0xFF;
Debug.Trace('RGB: ' + r + ',' + g + ',' + b)
//Get hex values
var hexchars="0123456789ABCDEF";
var r = hexchars.charAt((r >> 4) & 0xf) + hexchars.charAt(r & 0xF);
var g = hexchars.charAt((g >> 4) & 0xf) + hexchars.charAt(g & 0xF);
var b = hexchars.charAt((b >> 4) & 0xf) + hexchars.charAt(b & 0xF);
Debug.Trace('HEX: ' + r + g + b);
}
wtf thats not by matty, i made that
RE: How to make a struct? by Ezra on 08-01-2006 at 09:05 AM
quote: Originally posted by eljelly
quote: Originally posted by Ezra
Hmm, I guess you'll have to do that another way.
Here is some code that I think would help you
Color Picker Made By Matty:
code: //Show color picker common dialog (Made By Matty);
//Create our CHOOSECOLOR data block
var CHOOSECOLOR = Interop.Allocate(36);
CHOOSECOLOR.WriteDWORD(0, 36); //DWORD lStructSize
CHOOSECOLOR.WriteDWORD(4, 0); //HWND hwndOwner
CHOOSECOLOR.WriteDWORD(8, 0); //HWND hInstance
CHOOSECOLOR.WriteDWORD(12, 0x000000FF); //COLORREF rgbResult (COLORREF = 0x00bbggrr)
var CustColors = Interop.Allocate(64); //Create an array of 16 COLORREFs for CustColors
CHOOSECOLOR.WriteDWORD(16, CustColors.DataPtr); //COLORREF *lpCustColors (pointer to our array)
CHOOSECOLOR.WriteDWORD(20, 3); //DWORD Flags (3 = 2 (CC_FULLOPEN) + 1 (CC_RGBINIT) )
CHOOSECOLOR.WriteDWORD(24, 0); //LPARAM lCustData
CHOOSECOLOR.WriteDWORD(28, 0); //LPCCHOOKPROC lpfnHook
CHOOSECOLOR.WriteDWORD(32, 0); //LPCTSTR lpTemplateName
//Open the dialog box
var result = Interop.Call('comdlg32.dll', 'ChooseColorA', CHOOSECOLOR);
//If the user pressed ok convert it to hex
if(result == 1){
//Get decimal values
var r = CHOOSECOLOR.ReadDWORD(12) & 0xFF;
var g = (CHOOSECOLOR.ReadDWORD(12) / 0x100) & 0xFF;
var b = (CHOOSECOLOR.ReadDWORD(12) / 0x10000) & 0xFF;
Debug.Trace('RGB: ' + r + ',' + g + ',' + b)
//Get hex values
var hexchars="0123456789ABCDEF";
var r = hexchars.charAt((r >> 4) & 0xf) + hexchars.charAt(r & 0xF);
var g = hexchars.charAt((g >> 4) & 0xf) + hexchars.charAt(g & 0xF);
var b = hexchars.charAt((b >> 4) & 0xf) + hexchars.charAt(b & 0xF);
Debug.Trace('HEX: ' + r + g + b);
}
wtf thats not by matty, i made that 
OMG , sorry
RE: How to make a struct? by -=blu3+3y3s=- on 08-01-2006 at 06:17 PM
Thanks, guys for your answers. That works perfectly.
|