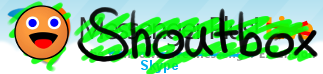
PHP login system help needed - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Skype & Technology (/forumdisplay.php?fid=9)
+---- Forum: Tech Talk (/forumdisplay.php?fid=17)
+----- Thread: PHP login system help needed (/showthread.php?tid=67277)
PHP login system help needed by Nathan on 10-13-2006 at 09:37 PM
Ok i am making a new login system and i dont know a lot about variables,
heres my login code:
code:
<?php
session_start();
ini_set ('display_errors', 1);
error_reporting (E_ALL & ~E_NOTICE);
if (isset ($_POST['submit'])) {
if ( (!empty ($_POST['username'])) && (!empty ($_POST['password'])) ) {
if ( ($_POST['username'] == 'moo') && ($_POST['password'] == 'meow') ) {
$_SESSION['Nathan'] = 'Nathan';
echo '<a href="<!--Link goes here-->">Go here</a>';
header ('Location: edit12345.php');
exit();
} else {
echo '<p>Sorry this is not the correct password or username, Go back and try again</p>';
}
} else {
echo '<p>Make sure password/username are correct</p>';
}
} else {
echo '<form action="index.php" method="post">
Username:<input type="text" name="username" size="20" /> <br />
Password:<input type="password" name="password" size="20" /> <br />
<input type="submit" name="submit" value="Log In" />
</form>';
}
?>
and I have tried loads of times to put sessions in there but i have failed
heres the page i want it to deny access to if theres no session/username is wrong.
code:
<?php
session_start();
ini_set ('display_errors', 1);
error_reporting (E_ALL & ~E_NOTICE);
if ($dbc = mysql_connect ('hostname', 'username', 'password')) {
if (!@mysql_select_db ('nathan1')) {
die ('<p>coud not select ' . mysql_error() . '</p>');
}
} else {
die ('<p>could not connect</p>');
}
$query = 'SELECT * FROM blog_entries ORDER
BY date_entered DESC';
if ($r = mysql_query ($query)) {
while ($row = mysql_fetch_array ($r)) {
echo "<h3 align='center'>{$row['title']}</h3><p>Date Entered: {$row['date_entered']}</p><div align='center' style='border: 1px solid white; width: 40%; margin-left: 25%;'>{$row['entry']}</div><br />
<div align='right' style='float: right;'><a href=\"edit_entry.php?id={$row['blog_id']}\">Edit</a></div>
<div align='left' style='float: left;'><a href=\"delete_entry.php?id={$row['blog_id']}\">Delete</a></div>
<hr color='black' />\n";
}
} else {
echo 'MOOOO';
}
mysql_close();
?>
So can you help please 
Ill be so greatful! Cheers.
Nathan
RE: A bit of help please :p by andrewdodd13 on 10-14-2006 at 09:43 AM
I think this is what you're looking for:
code: <?php
session_start();
if (!isset($_SESSION['Nathan']) && $_SESSION['Nathan'] != "Nathan")
die ("Not logged in");
ini_set ('display_errors', 1);
error_reporting (E_ALL & ~E_NOTICE);
if ($dbc = mysql_connect ('hostname', 'username', 'password')) {
if (!@mysql_select_db ('nathan1')) {
die ('<p>coud not select ' . mysql_error() . '</p>');
}
} else {
die ('<p>could not connect</p>');
}
$query = 'SELECT * FROM blog_entries ORDER
BY date_entered DESC';
if ($r = mysql_query ($query)) {
while ($row = mysql_fetch_array ($r)) {
echo "<h3 align='center'>{$row['title']}</h3><p>Date Entered: {$row['date_entered']}</p><div align='center' style='border: 1px solid white; width: 40%; margin-left: 25%;'>{$row['entry']}</div><br />
<div align='right' style='float: right;'><a href=\"edit_entry.php?id={$row['blog_id']}\">Edit</a></div>
<div align='left' style='float: left;'><a href=\"delete_entry.php?id={$row['blog_id']}\">Delete</a></div>
<hr color='black' />\n";
}
} else {
echo 'MOOOO';
}
mysql_close();
?>
RE: RE: A bit of help please :p by J-Thread on 10-14-2006 at 11:12 AM
quote: Originally posted by andrewdodd13
I think this is what you're looking for:
code: <?php
session_start();
if (empty($_SESSION['Nathan']) || $_SESSION['Nathan'] != "Nathan")
die ("Not logged in");
ini_set ('display_errors', 1);
error_reporting (E_ALL & ~E_NOTICE);
if ($dbc = mysql_connect ('hostname', 'username', 'password')) {
if (!@mysql_select_db ('nathan1')) {
die ('<p>coud not select ' . mysql_error() . '</p>');
}
} else {
die ('<p>could not connect</p>');
}
$query = 'SELECT * FROM blog_entries ORDER
BY date_entered DESC';
if ($r = mysql_query ($query)) {
while ($row = mysql_fetch_array ($r)) {
echo "<h3 align='center'>{$row['title']}</h3><p>Date Entered: {$row['date_entered']}</p><div align='center' style='border: 1px solid white; width: 40%; margin-left: 25%;'>{$row['entry']}</div><br />
<div align='right' style='float: right;'><a href=\"edit_entry.php?id={$row['blog_id']}\">Edit</a></div>
<div align='left' style='float: left;'><a href=\"delete_entry.php?id={$row['blog_id']}\">Delete</a></div>
<hr color='black' />\n";
}
} else {
echo 'MOOOO';
}
mysql_close();
?>
This is what you mean
RE: PHP login system help needed by -dt- on 10-14-2006 at 11:31 AM
quote: Originally posted by Nathan
error_reporting (E_ALL & ~E_NOTICE);
* -dt- slaps Nathan
real men use E_ALL
RE: RE: RE: A bit of help please :p by andrewdodd13 on 10-14-2006 at 12:58 PM
quote: Originally posted by J-Thread
quote: Originally posted by andrewdodd13
I think this is what you're looking for:
code: <?php
session_start();
if (empty($_SESSION['Nathan']) || $_SESSION['Nathan'] != "Nathan")
die ("Not logged in");
ini_set ('display_errors', 1);
error_reporting (E_ALL & ~E_NOTICE);
if ($dbc = mysql_connect ('hostname', 'username', 'password')) {
if (!@mysql_select_db ('nathan1')) {
die ('<p>coud not select ' . mysql_error() . '</p>');
}
} else {
die ('<p>could not connect</p>');
}
$query = 'SELECT * FROM blog_entries ORDER
BY date_entered DESC';
if ($r = mysql_query ($query)) {
while ($row = mysql_fetch_array ($r)) {
echo "<h3 align='center'>{$row['title']}</h3><p>Date Entered: {$row['date_entered']}</p><div align='center' style='border: 1px solid white; width: 40%; margin-left: 25%;'>{$row['entry']}</div><br />
<div align='right' style='float: right;'><a href=\"edit_entry.php?id={$row['blog_id']}\">Edit</a></div>
<div align='left' style='float: left;'><a href=\"delete_entry.php?id={$row['blog_id']}\">Delete</a></div>
<hr color='black' />\n";
}
} else {
echo 'MOOOO';
}
mysql_close();
?>
This is what you mean 
Sort of. I actually just forgot to close the bracket... the way I done it is because I always thought if the first part of an and statement was false the second part wasn't evaluated.
if (empty($_SESSION['Nathan']) || $_SESSION['Nathan'] != "Nathan")
This line is redundant. If the var is empty, it can't equal Nathan.
However, if $_SESSION['Nathan'] isn't set then you'll get a warning.
RE: PHP login system help needed by J-Thread on 10-14-2006 at 05:00 PM
You are wrong. My line isn't redundant at all.
If the var is empty, it can indeed not equal Nathan. When the var is empty, "empty($_SESSION['Nathan'])" will be true, so the other part of the line will not be evaluated.
However, if there is a value, "empty($_SESSION['Nathan'])" is false, and then it checks if "$_SESSION['Nathan'] != "Nathan"". When that is true, the $_SESSION['Nathan'] is appearantly not what we expected, en we should stop processing.
code: if (!isset($_SESSION['Nathan']) && $_SESSION['Nathan'] != "Nathan")
is really wrong (or, not what you meant). If the session var was not set, the first part will be true, so the second part will also be evaluated (to check if true && true). However, if it is not set it can never equal "Nathan".
Imagine:
$_SESSION['Nathan'] = "J-Thread";
Then "isset($_SESSION['Nathan'])" is true, so "!isset($_SESSION['Nathan'])" is false. Then the whole statement will be false, so the "die(...)" will not be executed. However, this was obviously not what you want!
RE: PHP login system help needed by Lou on 10-14-2006 at 05:27 PM
quote: Originally posted by andrewdodd13
if (!isset($_SESSION['Nathan']) && $_SESSION['Nathan'] != "Nathan")
die ("Not logged in");
That's not even correct. You never opened your if statement using { and never closed it using }
Curly braces are always needed in an if statement.
RE: PHP login system help needed by -dt- on 10-14-2006 at 05:36 PM
quote: Originally posted by .Lou
quote: Originally posted by andrewdodd13
if (!isset($_SESSION['Nathan']) && $_SESSION['Nathan'] != "Nathan")
die ("Not logged in");
That's not even correct. You never opened your if statement using { and never closed it using }
Curly braces are always needed in an if statement.
no they're not they're optional for single line use
RE: PHP login system help needed by J-Thread on 10-14-2006 at 06:21 PM
* J-Thread wonders why all those people think they can program...
Edit: That wasn't pointed to dt of course...
|