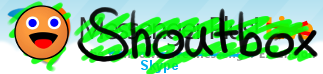
String manipulation in PHP - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Skype & Technology (/forumdisplay.php?fid=9)
+---- Forum: Tech Talk (/forumdisplay.php?fid=17)
+----- Thread: String manipulation in PHP (/showthread.php?tid=77986)
String manipulation in PHP by rav0 on 10-05-2007 at 12:50 PM
I'm trying to integrate an image gallery onto my website. I've chosen to temporarily use SPGM because it works without a database.
It's designed to integrate easily by just being included but it doesn't work with my website's (shoddy, homemade) friendly URL system.
Not being able to easily find what I need to modify in the SPGM code I found a different approach of buffering the output then modifying that (http://www.schilken.de/weblog/archives/2004/02/20/gallery-spgm-with-good-static-urls.htm is where my idea is from but it needs to be changed a bit).
I will have a string that is a snippet of HTML code. Inside that I need to find all links within the gallery and fix them like this:
"index.php?spgmGal=rav&spgmPic=7&title=something#spgmPicture" becomes
"/something?spgmGal=rav&spgmPic=7#spgmPicture" or even
"/something/rav/7#spgmPicture"
spgmGal and spgmPic won't always be there, and spgmPic won't be unless spgmGal is also. title will always be there (this is the heart of my friendly URLs and why the integration won't work as-is).
PS I have explained the plan I developed which I have trouble implementing because I don't know how to change the URL and I have come here to ask. If anybody knows of another way of fixing the problem of the invalid links being generated I would consider that also.
RE: String manipulation in PHP by hmaster on 10-05-2007 at 10:24 PM
This may not be the most efficient way to do it but it works.
code: <?php
$str = "index.php?spgmGal=rav&spgmPic=7&title=something#spgmPicture";
echo transformlink($str);
function transformlink($str) {
#get title
preg_match('/title=[A-Za-z0-9 ]+/', $str, $matches, PREG_OFFSET_CAPTURE);
$title = substr($matches[0][0], 6);
#check if spgmGal is there
if (preg_match("/spgmGal=[A-Za-z0-9 ]+/", $str)) {
preg_match('/spgmGal=[A-Za-z0-9 ]+/', $str, $matches, PREG_OFFSET_CAPTURE);
$spgmGal = substr($matches[0][0], 8);
} else {
$spgmGal = null;
}
#check if spgmPic is there
if(!is_null($spgmGal)) {
preg_match('/spgmPic=[A-Za-z0-9 ]+/', $str, $matches, PREG_OFFSET_CAPTURE);
$spgmPic = substr($matches[0][0], 8);
} else {
$spgmPic = null;
}
#get spgmPicture anchor
preg_match('/#[A-Za-z0-9 ]+/', $str, $matches, PREG_OFFSET_CAPTURE);
$spgmPicture = $matches[0][0];
#return transformed link
$thelink = (!is_null($spgmGal)) ? '/'.$title.'/'.$spgmGal.'/'.$spgmPic.$spgmPicture : '/'.$title.$spgmPicture;
return $thelink;
}
?>
RE: String manipulation in PHP by rav0 on 10-06-2007 at 02:35 AM
Thanks I've got that working to fix the URL properly.
Thing is, the input that I have is a chunk of HTML code, not just the URLs by themselves. I need to search for each URL and then fix it and replace it in the chunk of code. I think I could search for anything begining with ="index.php? and ending in a double quote but I don't know how to do it (as in <a href="index...">, <img src=").
|