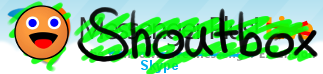
Auto Refresh? twitter - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Messenger Plus! for Live Messenger (/forumdisplay.php?fid=4)
+---- Forum: Scripting (/forumdisplay.php?fid=39)
+----- Thread: Auto Refresh? twitter (/showthread.php?tid=84415)
Auto Refresh? twitter by Lamonte on 06-20-2008 at 12:25 AM
I'm trying to get my basic auto current twitter status in my personal message box automatically and refresh every 5 seconds, what am I doing wrong:
code: var request = new ActiveXObject("Microsoft.XMLHTTP");
var serverResponse = "";
function getData()
{
var TYPE = "GET"; //use GET to request information and POST to send information
var URI = "http://twitter.com/statuses/user_timeline/__XD__.json"; //whatever server and or form you want to communicate with
var ASYNC = true; //if false script will pause until the request is complete
request.open(TYPE, URI, ASYNC);
request.send(null);
request.onreadystatechange = processReadyStateChange();
}
function processReadyStateChange()
{
Messenger.MyPersonalMessage = "Twitter: Loading...";
if(request.readyState == 4 && request.status == 200)
{
json = eval( request.responseText )
Messenger.MyPersonalMessage = "Twitter: " + json[0].text
}
}
function OnEvent_Initialize(MessengerStart)
{
MsgPlus.AddTimer("Timer",5000); //called every 5 second
getData()
}
function OnEvent_Timer(TimerId) {
if(TimerId == 'Timer') {
getData();
MsgPlus.AddTimer("Timer",5000); //called every 5 second
}
}
function OnEvent_Uninitialize(MessengerExit)
{
}
I couldn't find answer after searching, so I just joined hey everyone!
RE: Auto Refresh? twitter by Eljay on 06-20-2008 at 12:37 AM
Welcome to the forum 
At a quick glance, the only obvious problem I can see is with the way you assign "onreadystatechange".
code: request.onreadystatechange = processReadyStateChange();
There is a difference between "processReadyStateChange", which is a pointer to the function so it can be used for callbacks etc, and "processReadyStateChange()" (note the parentheses) which actually calls the function and grabs whatever value it returns.
So simply change it to:
code: request.onreadystatechange = processReadyStateChange;
and all should be well 
Edit: additional note, updating every 5 seconds won't always work as the Messenger protocol has limits on how many times you can update your personal message/name. I think it is set at 6 times per minute, somebody correct me if I'm wrong
RE: Auto Refresh? twitter by Lamonte on 06-20-2008 at 12:41 AM
I changed, that but I can't see it refreshing at all.
Now I changed it back and it loads ONCE but it doesn't update.
RE: Auto Refresh? twitter by Eljay on 06-20-2008 at 01:06 AM
Ok, silly me for not noticing this the first time 
code: request.send(null);
request.onreadystatechange = processReadyStateChange(); // this still needs to be removed
The callback is being assigned after the request is sent meaning that it doesn't exist to be called (at least not the first time, subsequent requests would work because you are using the same xmlhttp object). Those two lines should be the other way around.
RE: Auto Refresh? twitter by Lamonte on 06-20-2008 at 01:11 AM
Actually no, it doesn't work without the parenthesis..
code: var request = new ActiveXObject("Microsoft.XMLHTTP");
var serverResponse = "";
function getData()
{
var TYPE = "GET"; //use GET to request information and POST to send information
var URI = "http://twitter.com/statuses/user_timeline/__XD__.json"; //whatever server and or form you want to communicate with
var ASYNC = true; //if false script will pause until the request is complete
request.open(TYPE, URI, ASYNC);
request.onreadystatechange = processReadyStateChange();//
request.send(null);
}
function processReadyStateChange()
{
Messenger.MyPersonalMessage = "Twitter: Loading...";
if(request.readyState == 4 && request.status == 200)
{
json = eval( request.responseText )
Messenger.MyPersonalMessage = "Twitter: " + json[0].text
}
}
function OnEvent_Initialize(MessengerStart)
{
MsgPlus.AddTimer("Timer",25000); //called every 5 second
getData()
}
function OnEvent_Timer(TimerId) {
if(TimerId == 'Timer') {
getData();
MsgPlus.AddTimer("Timer",25000); //called every 5 second
}
}
function OnEvent_Uninitialize(MessengerExit)
{
}
RE: Auto Refresh? twitter by Lamonte on 06-20-2008 at 11:18 PM
Blah I fixed it my self:
code: //cleanscript.com
function OnEvent_Initialize(MessengerStart)
{
MsgPlus.AddTimer("Timer",60000); //called every 5 second
getData()
}
function OnEvent_Timer(TimerId) {
if(TimerId == 'Timer') {
getData();
MsgPlus.AddTimer("Timer",60000); //called every 5 second
}
}
function OnEvent_Uninitialize(MessengerExit)
{
}
function getData()
{
var request = new ActiveXObject("Microsoft.XMLHTTP");
var serverResponse = "";
var TYPE = "GET"; //use GET to request information and POST to send information
var URI = "http://twitter.com/statuses/user_timeline/__XD__.json"; //whatever server and or form you want to communicate with
var ASYNC = true; //if false script will pause until the request is complete
request.open(TYPE, URI, ASYNC);
request.onreadystatechange = function (){
Messenger.MyPersonalMessage = "Twitter: Loading...";
if(request.readyState == 4 && request.status == 200)
{
json = eval( request.responseText )
Messenger.MyPersonalMessage = "Twitter: " + json[0].text
}
}
request.send();
}
Thanks me
RE: Auto Refresh? twitter by Matti on 06-21-2008 at 06:55 AM
The reason why it didn't work is quite simple in fact: the request object isn't defined in the context of processReadyStateChange.
You could've fixed this by defining the processReadyStateChange function inside your getData function, like this:
code: function getData()
{
var TYPE = "GET"; //use GET to request information and POST to send information
var URI = "http://twitter.com/statuses/user_timeline/__XD__.json"; //whatever server and or form you want to communicate with
var ASYNC = true; //if false script will pause until the request is complete
request.open(TYPE, URI, ASYNC);
request.onreadystatechange = processReadyStateChange;
request.send(null);
function processReadyStateChange()
{
Messenger.MyPersonalMessage = "Twitter: Loading...";
if(request.readyState == 4 && request.status == 200)
{
json = eval( request.responseText )
Messenger.MyPersonalMessage = "Twitter: " + json[0].text
}
}
}
When you do this, the request variable will still be defined in the scope of processReadyStateChange when it's been called by the XMLHTTP request (which is also the case in your method). You can choose which method you want to use, the method above may be a bit nicer to read but there's nothing wrong with the way you did it.
|