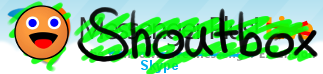
If statements and editing a script - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Messenger Plus! for Live Messenger (/forumdisplay.php?fid=4)
+---- Forum: Scripting (/forumdisplay.php?fid=39)
+----- Thread: If statements and editing a script (/showthread.php?tid=86097)
If statements and editing a script by vanillaflavoured on 09-22-2008 at 09:43 PM
Okay so I'm making some changes to a script (for personal reasons) which changes the first letter of a string to uppercase and adds a full stop to the end of the string.
This was all very well and if the string always had to end in a full stop but if I type something like "help!" the result would be "Help!." so I managed to get the script to, by default, add a full stop unless I added one myself or some other form of punctuation.
However my problem is I only want it to uppercase if there is actually a character a-z or A-Z as the first character as when I type Japanese characters it messes the first character up and if I try to send Messenger Plus commands like /busy or /all it creates problems with that too.
Soo yeah...any suggestions?
I've tried switches/case selects and if statements.
If I were able to code in VB/VBA then I'd be fine. Damn Javascript.
So yeah...answers on a postcard?
RE: If statements and editing a script by Jesus on 09-23-2008 at 01:58 AM
Actually, it's not javascript, it's Jscript (though they have many things in common).
As for your problem: If you can code in VB, you must have heard of regular expressions. They can also be used in Jscript, though I'm not sure how they handle the japanese characters.
RE: If statements and editing a script by Burningmace on 09-23-2008 at 11:47 PM
code: function startsWithAlpha(message) {
if(message.length == 0) { return false; }
var alpha = "qwertyuiopasdfghjklzxcvbnmQWERTYUIOPASDFGHJKLZXCVBNM";
var firstChar = message.charAt(0);
for(i = 0; i < alpha.length; i++) {
if(alpha.charAt == firstChar) {
return true;
}
}
return false;
}
This *should* work but I've not tested it and I've not coded in JS for ages.
RE: If statements and editing a script by roflmao456 on 09-24-2008 at 12:13 AM
quote: Originally posted by Burningmace
code: function startsWithAlpha(message) {
[...]
}
can be reduced to one line using regexp 
code: function startsWithAlpha(message){
return /^[A-Za-z]/.test(message);
}
i'm not a master of reg exp so it might have some errors.. lol 
quote: Originally posted by vanillaflavoured
Okay so I'm making some changes to a script (for personal reasons)
*cough*punctuator*cough* .
quote: However my problem is I only want it to uppercase if there is actually a character a-z or A-Z as the first character as when I type Japanese characters it messes the first character up and if I try to send Messenger Plus commands like /busy or /all it creates problems with that too.
the code above should fix that.
code: function OnEvent_ChatWndSendMessage(ChatWnd, Message){
if(startsWithAlpha(message)){
Message = "$" + Message.substr(1);
}
}
/*If I send 'LOL', it will output as '$OL'*/
RE: If statements and editing a script by Burningmace on 09-24-2008 at 12:46 AM
To uppercase the first character after you've detected that the fist character is indeed within the range a-Z you can do this:
code: message = message.charAt(0).toUpper() + message.substr(1);
RE: RE: If statements and editing a script by vanillaflavoured on 09-24-2008 at 12:20 PM
quote: Originally posted by Burningmace
To uppercase the first character after you've detected that the fist character is indeed within the range a-Z you can do this:
code: message = message.charAt(0).toUpper() + message.substr(1);
This bit I had already sorted out.
Basically, here's what I already have and yes I know this all could probably be done MUCH more simply but like I said, I normally program databases in VB so I have NO experience with JScript etc.
code: function OnEvent_ChatWndSendMessage(pChatWnd, Message){
var endChar = Message.charAt(Message.length-1)
switch (endChar)
{
case "!":
endChar = ""
break;
case "?":
endChar = ""
break;
case ".":
endChar = ""
break;
case ")":
endChar = ""
break;
case ":":
endChar = ""
break;
default:
endChar = "."
}
Message = Message.substr(0,1).toUpperCase() + Message.substr(1) + endChar
return Message;
}
RE: If statements and editing a script by roflmao456 on 09-24-2008 at 07:55 PM
quote: Originally posted by vanillaflavoured
code: function OnEvent_ChatWndSendMessage(pChatWnd, Message){
[...]
}
shortened:
code: function OnEvent_ChatWndSendMessage(ChatWnd, Message){
if(/[a-z\d]$/i.test(Message)) Message += ".";
return Message.charAt(0).toUpperCase() + Message.substr(1);
}
RE: If statements and editing a script by vanillaflavoured on 09-25-2008 at 10:41 AM
It's still not letting me use the Messenger Plus command though.
However it will let me use custom commands introduced by other scripts. I just can't use the default ones...
I'm not sure if it's directly related to this script I'm trying to write though but if I deactivate it, it starts working then...soo...
RE: If statements and editing a script by Jesus on 09-25-2008 at 10:53 AM
That's because the code roflmao456 posted also adds a full stop to any commands you type. This should work:
code: function OnEvent_ChatWndSendMessage(ChatWnd, Message){
if(/[a-z\d]$/i.test(Message) && Message.charAt(0) != "/") Message += ".";
return Message.charAt(0).toUpperCase() + Message.substr(1);
}
RE: If statements and editing a script by vanillaflavoured on 09-25-2008 at 11:22 AM
Cheers guys. Think that's done it. Everything seems to be working great.
|