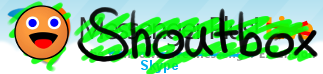
Help - I'm new to scripting! - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Messenger Plus! for Live Messenger (/forumdisplay.php?fid=4)
+---- Forum: Scripting (/forumdisplay.php?fid=39)
+----- Thread: Help - I'm new to scripting! (/showthread.php?tid=87276)
Help - I'm new to scripting! by whiz on 11-15-2008 at 03:12 PM
I've just started working on an "Instant Response" script, that can send a message or a /command after someone says something to you. But I have a few problems since I am very new to this:
1) I don't know how to make an enable/disable option (for chat windows and the contact list) in the script option menu.
2) I don't know how to make an options screen, to change the delay and message, either!
If anyone can give me the basic code for it, I would really appreciate it. 
I have attached the script file in the message below, and as an attachment (in script format - ".js").
JScript code:
var SettingMessage;
var SettingTimer;
function OnEvent_Initialize(MessengerStart)
{
SettingMessage = "/nudge";
SettingTimer = 100;
}
function OnEvent_Timer(TimerID)
{
Debug.Trace("Timer launched");
Messenger.OpenChat(TimerID).SendMessage(SettingMessage);
}
function OnEvent_ChatWndReceiveMessage(ChatWnd, Origin, Message, MessageKind)
{
if (Origin != Messenger.MyName) {
Debug.Trace("Message received");
var Contacts = ChatWnd.Contacts;
var e = new Enumerator(Contacts);
for(; !e.atEnd(); e.moveNext()) {
var Contact = e.item();
Debug.Trace(" " + Contact.Email);
}
if (Message != SettingMessage)
{
var Contacts = ChatWnd.Contacts;
var e = new Enumerator(Contacts);
var NoContacts = 0;
for(; !e.atEnd(); e.moveNext()) {
var Contact = e.item();
Debug.Trace(" " + Contact.Email);
NoContacts = NoContacts + 1;
}
Debug.Trace(NoContacts);
if (NoContacts == 1) {
MsgPlus.AddTimer(Contact.Email, SettingTimer);
Debug.Trace("Timer started");
}
}
}
}
RE: Help - I'm new to scripting! by matty on 11-25-2008 at 04:31 PM
For the menu option look at something like this
Volv's reply to Tips
As far as creating a Window this is all done in XML. Take a look at some other script and see how they do things and don't forget to check out the official documentation on the site.
RE: Help - I'm new to scripting! by whiz on 11-26-2008 at 08:16 PM
Okay, I get the menu, but how can I use the menu to enable and disable the script?
RE: Help - I'm new to scripting! by matty on 11-26-2008 at 08:23 PM
Uusing this: JScript code:
function OnEvent_MenuClicked(sMenuId){
if(sMenuId=="mnuItem1"){
...
}
}
You can set a boolean variable that will enable or disable the script.
RE: Help - I'm new to scripting! by whiz on 11-26-2008 at 09:01 PM
I get that, but I'm still not sure how to make a boolean variable to enable/disable the script... (sorry, I'm really new to this).
RE: Help - I'm new to scripting! by matty on 11-27-2008 at 02:29 PM
Do you want the ability to prevent nudging if the script is "disabled" is that the affect you are trying to achieve?
Also I know you are new but you can cut down on some code for example:
JScript code:
// Create an object to store our sent messages
var mySentMessages = {};
var bEnabled = true;
function OnEvent_ChatWndReceiveMessage(ChatWnd, Origin, Message, MessageKind) {
if (mySendMessages[ChatWnd.Handle] != Message) {
Debug.Trace("Message received");
if (Message != '/nudge') {
if (ChatWnd.Contacts.Count == 1 && bEnabled == true) {
for (var e = new Enumerator(ChatWnd.Contacts); !e.atEnd(); e.moveNext()) {
// store the new chat window in a variable
var pChat = Messenger.OpenChat(e.item().Email)
// check if the chat window's typing area is enabled and the user isn't blocked
if (pChat.EditChangeAllowed == true && e.item().Blocked == false) {
pChat.SendMessage('/nudge');
}
}
}
}
}
}
function OnEvent_ChatWndSendMessage(ChatWnd, Message){
mySentMessages[ChatWnd.Handle] = Message; // store the last sent message in an object
}
function OnEvent_ChatWndDestroyed(ChatWnd) {
delete mySentMessages[ChatWnd.Handle]; // delete the message from the object as it isn't needed anymore
}
/*
Use bEnabled to control whether or not you want the nudge to be sent.
When the user clicks the menu item to disable the script set bEnabled = false; and vice versa when they are enabling it.
*/
RE: Help - I'm new to scripting! by whiz on 11-27-2008 at 07:37 PM
So, in the "on click" thing for enable and disable in the script menu, I need to put "bEnabled = [true/false]", right?
RE: Help - I'm new to scripting! by matty on 11-27-2008 at 07:38 PM
quote: Originally posted by whiz
So, in the "on click" thing for enable and disable in the script menu, I need to put "bEnabled = [true/false]", right?
Yeah exactly. You want to change the variable so that it will disable the script internally.
RE: Help - I'm new to scripting! by whiz on 12-07-2008 at 11:25 AM
Sorry - another question!
I'm trying this code on a simpler script, that displays toasts to the user regarding certain information, like sign-ins, name changes and so on.
Am I right in thinking that the menu items should be set like this:
JScript code:
function OnEvent_MenuClicked(sMenuId)
{
if(sMenuId=="Enable")
{
var bEnabled = true;
var Message = "Toaster has just been enabled!";
Message = MsgPlus.RemoveFormatCodes(Message);
MsgPlus.DisplayToast("Toaster", Message);
Debug.Trace("Toaster | Enable");
}
if(sMenuId=="Disable")
{
var bEnabled = false;
var Message = "Toaster has just been disabled!";
Message = MsgPlus.RemoveFormatCodes(Message);
MsgPlus.DisplayToast("Toaster", Message);
Debug.Trace("Toaster | Disable");
}
}
And then each toast should be like this:
JScript code:
function OnEvent_Signin(Email)
{
if(bEnabled=="true")
{
var Message = "You have been signed in.";
Message = MsgPlus.RemoveFormatCodes(Message);
MsgPlus.DisplayToast("Toaster", Message);
Debug.Trace("Toaster | UserSign-in");
}
}
RE: Help - I'm new to scripting! by Matti on 12-07-2008 at 11:29 AM
That'll work, but not in the way you'd expect it. Therefore, in your OnEvent_Signin function, use
JScript code:
if(bEnabled)
since the way you do it now is by comparing it to the literal message "true" rather than the boolean value true.
RE: RE: Help - I'm new to scripting! by NanoChromatic on 12-07-2008 at 11:36 AM
quote: Originally posted by Matti
That'll work, but not in the way you'd expect it. Therefore, in your OnEvent_Signin function, use
JScript code:
if(bEnabled)
since the way you do it now is by comparing it to the literal message "true" rather than the boolean value true. 
Wow. I'm learning Pascal For Soldat.
Although I'm first going to try some nice scripts with WLM.
How would i start or is there a website that can help me?
Thx.
RE: Help - I'm new to scripting! by Matti on 12-07-2008 at 12:26 PM
quote: Originally posted by NanoChromatic
Wow. I'm learning Pascal For Soldat.
Why is that Pascal? It's just a regular boolean check: "if bEnabled (equals true), then do this."
quote: Originally posted by NanoChromatic
Although I'm first going to try some nice scripts with WLM.
How would i start or is there a website that can help me?
Well, there are no real step-by-step tutorials on how to make script, since every script is different. There are some good resources like mpscripts.net. But remember that the first step in learning Plus! Live scripting is by having a look at the Scripting Documentation.
RE: RE: Help - I'm new to scripting! by NanoChromatic on 12-07-2008 at 02:08 PM
quote: Originally posted by Matti
quote: Originally posted by NanoChromatic
Wow. I'm learning Pascal For Soldat.
Why is that Pascal? It's just a regular boolean check: "if bEnabled (equals true), then do this."
quote: Originally posted by NanoChromatic
Although I'm first going to try some nice scripts with WLM.
How would i start or is there a website that can help me?
Well, there are no real step-by-step tutorials on how to make script, since every script is different. There are some good resources like mpscripts.net. But remember that the first step in learning Plus! Live scripting is by having a look at the Scripting Documentation.
I Didnt mean Thats pascal XD
I Just thought i would say im learning pascal 
Haha, Anyway Thanks .
RE: Help - I'm new to scripting! by whiz on 12-07-2008 at 04:06 PM
Okay, I've now got this new sign-in code:
JScript code:
function OnEvent_Signin(Email)
{
if(bEnabled)
{
var Message = "You have been signed in.";
Message = MsgPlus.RemoveFormatCodes(Message);
MsgPlus.DisplayToast("Toaster", Message);
Debug.Trace("Toaster | UserSign-in [On]");
}
else
{
Debug.Trace("Toaster | UserSign-in [Off]");
}
}
But whether the script is "enabled" or "disabled" (as set in the script menu), it always comes up! Any ideas?
RE: RE: Help - I'm new to scripting! by Jesus on 12-08-2008 at 12:39 AM
quote: Originally posted by whiz
Okay, I've now got this new sign-in code:
code: ...
But whether the script is "enabled" or "disabled" (as set in the script menu), it always comes up! Any ideas?
That's because of the way the menu function you posted earlier was coded.
JScript code:
function OnEvent_MenuClicked(sMenuId)
{
if(sMenuId=="Enable")
{
[b]var[/b] bEnabled = true; var Message = "Toaster has just been enabled!";
Message = MsgPlus.RemoveFormatCodes(Message);
MsgPlus.DisplayToast("Toaster", Message);
Debug.Trace("Toaster | Enable");
}
if(sMenuId=="Disable")
{
[b]var[/b] bEnabled = false; var Message = "Toaster has just been disabled!";
Message = MsgPlus.RemoveFormatCodes(Message);
MsgPlus.DisplayToast("Toaster", Message);
Debug.Trace("Toaster | Disable");
}
}
The bolded var statements redeclare the bEnabled variable as a local variable, so their values aren't saved to the global variable.
In other words; this menu function does not change the script's status in any way.
Remove both bolded "var"s (so the lines say "bEnabled = true/false;") and you should be fine.
RE: Help - I'm new to scripting! by whiz on 12-08-2008 at 07:49 PM
It works! Thanks!
|