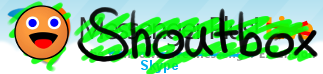
Read Text from a file - Printable Version
-Shoutbox (https://shoutbox.menthix.net)
+-- Forum: MsgHelp Archive (/forumdisplay.php?fid=58)
+--- Forum: Messenger Plus! for Live Messenger (/forumdisplay.php?fid=4)
+---- Forum: Scripting (/forumdisplay.php?fid=39)
+----- Thread: Read Text from a file (/showthread.php?tid=95798)
Read Text from a file by TehGoatLord on 11-07-2010 at 02:51 AM
Alright, so I need a script that reads a specific line from a specific file and then parse it and output the parsed text onto the current chat window.
Say it reads line 255 from a text file
Then it removes a certain string from the line
and outputs it!
If it helps anyone, I already did this in mIRC Scripting
code: $read(C:\Program Files\AssaultCube_v1.1.0.3\config\saved.cfg, 255)
That's basically what I want, the full function I created is:
code: alias ACServ {
//say /connect $remove($remove($read(C:\Program Files\AssaultCube_v1.1.0.3\config\saved.cfg, 255), alias "serverinfo" [), ])
}
I'm sorry if I'm unclear
RE: Read Text from a file by matty on 11-07-2010 at 03:40 AM
Here are 2 untested options to get get you started.
Javascript code:
function ReadLineFromFile(sFile, nLine) {
var f = new ActiveXObject("Scripting.FileSystemObject").OpenTextFile(sFile, 1 /* ForReading */);
while (--nLine > 0 && !f.AtEndOfStream) {
fso.ReadLine();
}
var s = fso.ReadLine();
f.Close();
return s;
}
Javascript code:
function ReadLineFromFile(sFile, nLine) {
var f = new ActiveXObject("Scripting.FileSystemObject").OpenTextFile(sFile, 1 /* ForReading */);
var s = f.ReadAll();
f.Close()
var FileContents = s.split('\r\n');
return FileConents(nLine-1);
}
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 04:47 AM
Sorry but how do I implement it ?
RE: Read Text from a file by Matti on 11-07-2010 at 10:11 AM
matty just provided you with two possible implementations of how you could read a single line from a file (what's in a function name?). Basically, you copy one of those functions in your script and call them:
Javascript code:
var line = ReadLineFromFile("C:\\Program Files\\AssaultCube_v1.1.0.3\\config\\saved.cfg", 255);
// line now contains the 255th line from saved.cfg
Of course, the most important part is when you call the function and what you do with it. If I understand correctly, you want to create a new chat command "/acserv" which will read the line, do some modifications with it and then output it as a chat message.
- In order to make your script do something when a chat message is sent by the current user, you'll need to create an OnEvent_ChatWndSendMessage function. This function will be called whenever a message is sent, allowing you to modify it before it gets sent. Have a look at the scripting documentation on how to define this event in your script.
- Inside this function, you'll want to check whether the message starts with your command "/acserv". There are a lot of cases which you need to take into account when parsing script commands, for example when there is more than one slash before a command, that command should be escaped and thus not parsed at all. Also, there are some limitations on which characters are allowed in the command name and the parameters. Luckily, CookieRevised wrote a regular expression which deals with all these cases.
Javascript code:
var m;
if ( m = /^\/([^ \n\r\v\xA0\/][^ \n\r\v\xA0]*)[\s\xA0]?([\s\S]*)/.exec(sMessage) ) {
var command = m[1].toLowerCase();
var parameter = m[2];
if ( command == 'acserv' ) {
// do something and return a modified message
}
}
return sMessage;
Source: CookieRevised's reply to Gettin data from "/" commands (small modifications by myself)
- Inside the command parser check if ( command == 'acserv' ) { ... }, you place your actual code. That is, call ReadLineFromFile, remove something from the returned string and output it by returning it back to the event function. So you start off with the first code block in this post, then do some replace() on the string, such as:
Javascript code:
line = line.replace('alias "serverinfo [', '');
line = line.replace(']', '');
and finally return line;.
If you're planning to use the second function matty posted, you'll want to fix a few things in his code:
Javascript code:
function ReadLineFromFile(sFile, nLine) {
var f = new ActiveXObject("Scripting.FileSystemObject").OpenTextFile(sFile, 1 /* ForReading */);
var s = f.ReadAll();
f.Close(); // added semicolon
var FileContents = s.split(/\r?\n/); // matches both \r\n and \n
return FileContents[nLine-1]; // fixed variable name and needs [ ] instead of ( ) since it's an array accessor.
}
I can't tell which of those implementations is the best, they all look pretty good. In most cases, the first method will be faster since it won't have to read the whole file, yet the second method may be easier to understand.
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 02:02 PM
This is what I did.. But the command isn't recognized D:
code: function ReadLineFromFile(sFile, nLine) {
var f = new ActiveXObject("Scripting.FileSystemObject").OpenTextFile(sFile, 1 /* ForReading */);
var s = f.ReadAll();
f.Close()
var FileContents = s.split('\r\n');
return FileConents(nLine-1);
}
function OnEvent_ChatWndSendMessage() {
var m;
if ( m = /^\/([^ \n\r\v\xA0\/][^ \n\r\v\xA0]*)[\s\xA0]?([\s\S]*)/.exec(sMessage) ) {
var command = m[1].toLowerCase();
var parameter = m[2];
if ( command == 'acserv' ) {
var line = ReadLineFromFile("C:\\Program Files\\AssaultCube_v1.1.0.3\\config\\saved.cfg", 255);
// line now contains the 255th line from saved.cfg
line = line.replace('alias "serverinfo [', '');
line = line.replace(']', '');
}
}
return sMessage;
}
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 02:04 PM
I'm having problem using the event o_o
RE: Read Text from a file by matty on 11-07-2010 at 02:10 PM
Well first you are doing it wrong. You need to use the OnEvent_ChatWndSendMessage() function. Have a look at the official documents.
Also I made a mistake in my code and the last return line should use [] not ().
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 04:47 PM
I find the official documents rather confusing it doesn't have examples and stuff so it's rather hard to figure out and I wont say that I'm good in English either. It'd be a great help if someone would create the script for me
RE: Read Text from a file by whiz on 11-07-2010 at 05:24 PM
You need to include the parameters (ChatWnd and Message) for OnEvent_ChatWndReceiveMessage().
JScript code:
function ReadLineFromFile(sFile, nLine)
{
var f = new ActiveXObject("Scripting.FileSystemObject").OpenTextFile(sFile, 1 /* ForReading */);
var s = f.ReadAll();
f.Close()
var FileContents = s.split('\r\n');
return FileConents[nLine - 1];
}
function OnEvent_ChatWndSendMessage(oChatWnd, sMessage)
{
var m;
if (m = /^\/([^ \n\r\v\xA0\/][^ \n\r\v\xA0]*)[\s\xA0]?([\s\S]*)/.exec(sMessage))
{
var command = m[1].toLowerCase();
var parameter = m[2];
if (command == 'acserv')
{
var line = ReadLineFromFile("C:\\Program Files\\AssaultCube_v1.1.0.3\\config\\saved.cfg", 255);
// line now contains the 255th line from saved.cfg
line = line.replace('alias "serverinfo [', '');
line = line.replace(']', '');
}
}
return sMessage;
}
If you're not sure how to put together and compile the script, have a look at the attachment.
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 06:09 PM
It doesn't work!
"The command you entered was not recognized
if the message was not meant to be a command etc"
RE: Read Text from a file by matty on 11-07-2010 at 06:30 PM
The highlighted line was forgotten.
JScript code:
function ReadLineFromFile(sFile, nLine)
{
var f = new ActiveXObject("Scripting.FileSystemObject").OpenTextFile(sFile, 1 /* ForReading */);
var s = f.ReadAll();
f.Close()
var FileContents = s.split('\r\n');
return FileConents[nLine - 1];
}
function OnEvent_ChatWndSendMessage(oChatWnd, sMessage)
{
var m;
if (m = /^\/([^ \n\r\v\xA0\/][^ \n\r\v\xA0]*)[\s\xA0]?([\s\S]*)/.exec(sMessage))
{
var command = m[1].toLowerCase();
var parameter = m[2];
if (command == 'acserv')
{
var line = ReadLineFromFile("C:\\Program Files\\AssaultCube_v1.1.0.3\\config\\saved.cfg", 255);
// line now contains the 255th line from saved.cfg
line = line.replace('alias "serverinfo [', '');
line = line.replace(']', '');
return line; }
}
return sMessage;
}
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 06:48 PM
Nope. It's still not working
RE: Read Text from a file by Matti on 11-07-2010 at 07:42 PM
You guys are still using the bugged version of ReadLineFromFile(). On the last line, "FileContents" is misspelled, which I already mentioned in my previous post.
Another possible issue could be that the file you're trying to read is saved as Unicode. By default, OpenTextFile reads the file as ASCII so you need to pass in an extra parameter to override this behavior. Therefore, I added an extra parameter to the function which takes care of this. You could try to detect whether a file is saved as ASCII or Unicode, but that requires some Win32 trickery.
Javascript code:
function ReadLineFromFile(sFile, nLine, bUnicode) {
var f = new ActiveXObject("Scripting.FileSystemObject").OpenTextFile(sFile, 1 /* ForReading */, false, (bUnicode ? -1 : 0));
var s = f.ReadAll();
f.Close();
var FileContents = s.split(/\r?\n/);
if(nLine > FileContents.length) return false; // implement some error handling here?
return FileContents[nLine-1];
}
function OnEvent_ChatWndSendMessage(oChatWnd, sMessage) {
var m;
if (m = /^\/([^ \n\r\v\xA0\/][^ \n\r\v\xA0]*)[\s\xA0]?([\s\S]*)/.exec(sMessage)) {
var command = m[1].toLowerCase();
var parameter = m[2];
if (command == 'acserv') {
var line = ReadLineFromFile("C:\\Program Files\\AssaultCube_v1.1.0.3\\config\\saved.cfg", 255, false); // change false to true if Unicode
// line now contains the 255th line from saved.cfg
line = line.replace('alias "serverinfo [', '');
line = line.replace(']', '');
return line;
}
}
return sMessage;
}
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 08:01 PM
Not workin, man ._. I don't the MSGPlus can call the function at all.
/acserv
doesn't work.
RE: Read Text from a file by matty on 11-07-2010 at 08:07 PM
What version of Windows Live Messenger do you have?
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 08:14 PM
14.0.8117.416
RE: Read Text from a file by Matti on 11-07-2010 at 08:22 PM
Could you be a bit more specific about what exactly isn't working? When I run the last snippet I posted, it works flawlessly and I'm on the same version of Messenger as well (WLM 2009).
- Are you sure you saved your script files as Unicode (UCS-2 Little Endian)?
- Does the script debugger window report any errors?
- Have you tried debugging it by adding some Debug.Trace() lines to see what the value of the variables are at certain points?
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 08:30 PM
![[Image: acservmsnfault.JPG]](http://www.rantages.com/acservmsnfault.JPG)
There it is
I'm a pretty much noob at this debugging and stuff ._. what exactly do I need to do starting from the beginning?
RE: Read Text from a file by Matti on 11-07-2010 at 08:42 PM
Where are you pasting the snippets into? Are you using the built-in script editor of Plus! Live? If so, does it say "Script has successfully started" in the debugger when you click "Save All"?
What exactly is at line 255 of that saved.cfg file of yours? I see you were doing something with /connect for your mIRC script, perhaps it fails because the line read from the file starts with a slash?
With debugging, I mean trying to get an idea about what state the script is in at certain points during execution. For example, it might be interesting to place a Debug.Trace call just after the command parser, to check whether the command is correctly detected:
Javascript code:
// ...
if (command == 'acserv') {
Debug.Trace('Found command /acserv');
var line = ReadLineFromFile( //...
Another interesting value to check is what is sent to the chat window. Just before the return statement, you could throw in a Debug.Trace call and see what the value of line is.
Javascript code:
line = line.replace(']', '');
Debug.Trace('line = '+line);
return line;
These are just some examples of how one could find out what's happening inside the script. Ultimately, you should be able to pinpoint the location where something doesn't go as planned and to fix the problem. And that is how we program stuff, through trial and error.
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 08:51 PM
This is what the script says:
code: Function called: OnEvent_ChatWndSendMessage
Function called: OnEvent_ChatWndSendMessage
Error: Object doesn't support this property or method (code: -2146827850)
File: AssaultCube Read.js. Line: 18.
Function OnEvent_ChatWndSendMessage returned an error. Code: -2147352567
and this is the cfg file
http://www.rantages.com/saved.cfg
Also, the connect is added later on in my mIRC alias.
RE: Read Text from a file by Matti on 11-07-2010 at 10:36 PM
Now that's a useful error message! 
Line 18 is:
Javascript code:
line = line.replace('alias "serverinfo [', '');
This is just weird. I tried this myself and indeed, ReadLineFromFile returns false, meaning that the requested line number exceeds the amount of lines in the file. Apparently, the empty lines were omitted from the line array when doing the split(). However, when I replaced the regular expression by a normal string, it correctly produced an array with empty strings for empty lines! It appears that this is an inconsistency in the JScript implementation itself (thanks for that, Microsoft), so the only way to get around this is by using a string as separator or by writing your own split() method. In this case, using a string will do. 
Just replace line 5 with:
Javascript code:
var FileContents = s.split("\n");
and it should work. In order to prevent further problems with this, you could add some error handling in case ReadLineFromFile returns false:
Javascript code:
var line = ReadLineFromFile("C:\\Program Files\\AssaultCube_v1.1.0.3\\config\\saved.cfg", 255, false);
if( line === false ) return ''; // line not found, die silently
line = line.replace('alias "serverinfo" [', '');
// ...
RE: Read Text from a file by TehGoatLord on 11-07-2010 at 10:45 PM
Thanks!
This works perfectly!
Thank you so much for the help, guys
|