[C++ Tutorial] Events and the Plus API |
Author: |
Message: |
RaceProUK
Elite Member
    

Posts: 6073 Reputation: 57
39 / / 
Joined: Oct 2003
|
O.P. [C++ Tutorial] Events and the Plus API
MSNFanatic has sample code for Messenger events, but it's needlessly complicated in my view, and doesn't really hint as to how to achieve the same effect in Plus. So, I'm writing this tutorial to take developers through step-by-step.
First of all, copy the header and CPP code below into separate files.
Second, in Initialize(), either typecast (quick and dirty) or use QueryInterface() (sensible but long-winded) the iMessengerObj pointer to IMessenger3*. Save this in a global variable.
Third, create a new MessengerEvents object, saving that in a global variable as well.
Fourth, and last, fill in the Invoke() function in MessengerEvents.cpp with your code.
Easy when you know how
NB: Remember to delete the MessengerEvents pointer in Uninitialize()!
Header:
code: // I think you can see where this is all used 
class MessengerEvents : public IDispatch {
public:
MessengerEvents();
~MessengerEvents();
STDMETHODIMP QueryInterface(REFIID iid, void **obj);
ULONG STDMETHODCALLTYPE AddRef();
ULONG STDMETHODCALLTYPE Release();
HRESULT STDMETHODCALLTYPE GetTypeInfoCount(unsigned int *info);
HRESULT STDMETHODCALLTYPE GetTypeInfo(unsigned int info, LCID lcid, ITypeInfo **iTInfo);
HRESULT STDMETHODCALLTYPE GetIDsOfNames(REFIID iid, OLECHAR **names, unsigned int cNames, LCID lcid, DISPID *dispId);
HRESULT STDMETHODCALLTYPE Invoke(DISPID dispIDMember, REFIID iid, LCID lcid, WORD flags, DISPPARAMS *params, VARIANT *result, EXCEPINFO *excepInfo, unsigned int *argErr);
private:
IConnectionPoint *sink;
DWORD cookie;
int refCount;
};
CPP:
code: #include <windows.h>
// Part of the Messenger API package
#include "msgrua.h"
#include "msgruaid.h"
// Rename this to match the name of the variable in the file with
Initialize()
extern IMessenger3 *MSN;
// COnnects to Messenger to provide an event sink
MessengerEvents::MessengerEvents() {
IConnectionPointContainer *sinkBox;
refCount = 1;
// Ask MSN for a collection of event interfaces
HRESULT hr =
MSN->QueryInterface(IID_IConnectionPointContainer, (void**)&sinkBox);
cookie = 0;
if (SUCCEEDED(hr)) {
// Ask the collection for the interface we want
(DMessengerEvents)
hr =
sinkBox->FindConnectionPoint(DIID_DMessengerEvents, &sink);
if (SUCCEEDED(hr))
// Tell Messenger we want to intercept events
sink->Advise(this, &cookie);
if (sinkBox) sinkBox->Release();
}
}
MessengerEvents::~MessengerEvents() {
// Tell Messenger we no longer want to intercept events
sink->Unadvise(cookie);
sink->Release();
}
// Don't worry too much about this one: it's here so we can actually
compile
STDMETHODIMP MessengerEvents::QueryInterface(REFIID iid, void **obj)
{
if (obj == NULL) return E_INVALIDARG;
*obj = NULL;
if (iid == IID_IUnknown) *obj = (IUnknown*)this;
if (iid == DIID_DMessengerEvents || iid == IID_IDispatch)
*obj = (IDispatch*)this;
if (*obj == NULL) return E_NOINTERFACE;
AddRef();
return S_OK;
}
ULONG STDMETHODCALLTYPE MessengerEvents::AddRef() {
return ++refCount;
}
ULONG STDMETHODCALLTYPE MessengerEvents::Release() {
return --refCount;
}
HRESULT STDMETHODCALLTYPE MessengerEvents::GetTypeInfoCount(unsigned
int *info) {
return E_NOTIMPL;
}
HRESULT STDMETHODCALLTYPE MessengerEvents::GetTypeInfo(unsigned int
info, LCID lcid, ITypeInfo **iTInfo) {
return E_NOTIMPL;
}
HRESULT STDMETHODCALLTYPE MessengerEvents::GetIDsOfNames(REFIID iid,
OLECHAR **names, unsigned int cNames, LCID lcid, DISPID *dispId) {
return E_NOTIMPL;
}
// OK, this is the most important part. Use 'msgruaid.h' to find the
event codes
HRESULT STDMETHODCALLTYPE MessengerEvents::Invoke(DISPID event,
REFIID iid, LCID lcid, WORD flags, DISPPARAMS *params, VARIANT
*result, EXCEPINFO *excepInfo, unsigned int *argErr) {
if (!params)
return E_INVALIDARG;
switch (event) {
// Put here your 'case' statements, one per event
}
return S_OK;
}
Get the Messenger API stuff - the .h files need to be placed with the files above, and the .lib file needs to be there too, as well as listed to be linked to.
This post was edited on 03-01-2005 at 01:28 PM by RaceProUK.
|
|
03-01-2005 01:27 PM |
|
 |
TheBlasphemer
Senior Member
   
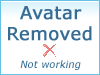
Posts: 714 Reputation: 47
36 / – / –
Joined: Mar 2004
|
RE: [C++ Tutorial] Events and the Plus API
For more information about how Invoke works:
http://msdn.microsoft.com/library/default.asp?url...htm/chap5_61id.asp
Please keep in mind that the arguments you get passed trough params are IN REVERSE ORDER!
Another trick to make it easier is to save the typelibrary of the DMessengerEvents object,
then make a class that has DMessengerEvents as public parent,
then on creation load the typelibrary using CreateTypeLib, and use ITypeLib::GetTypeInfo to get the type info.
then pass on all Invoke and GetIDsByNames etc on to that ITypeInfo, and you can just use the simple functions of the class instead of implementing the dodgy Invoke call 
|
|
03-01-2005 01:35 PM |
|
 |
Dempsey
Scripting Contest Winner
    

http://AdamDempsey.net
Posts: 2395 Reputation: 53
37 / / 
Joined: Jul 2003
|
RE: [C++ Tutorial] Events and the Plus API
great tutorial raceprouk  i was looking forward to this since you mentioned it on your blog 
|
|
03-01-2005 07:48 PM |
|
 |
RaceProUK
Elite Member
    

Posts: 6073 Reputation: 57
39 / / 
Joined: Oct 2003
|
O.P. RE: [C++ Tutorial] Events and the Plus API
Once I get my new site done and up, I may add a tutorials section to it, with this being one of them.
|
|
03-02-2005 08:11 AM |
|
 |
TheBlasphemer
Senior Member
   
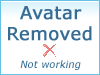
Posts: 714 Reputation: 47
36 / – / –
Joined: Mar 2004
|
RE: [C++ Tutorial] Events and the Plus API
quote: Originally posted by raceprouk
Once I get my new site done and up, I may add a tutorials section to it, with this being one of them.
I'm having the same idea, but I forget it everytime I have some spare time left 
|
|
03-02-2005 11:44 AM |
|
 |
RaceProUK
Elite Member
    

Posts: 6073 Reputation: 57
39 / / 
Joined: Oct 2003
|
O.P. RE: [C++ Tutorial] Events and the Plus API
You should: I'm sure they'll go down a treat
EDIT: In fact, a Wiki may be worth the effort...
This post was edited on 03-02-2005 at 09:52 PM by RaceProUK.
|
|
03-02-2005 09:51 PM |
|
 |
Dempsey
Scripting Contest Winner
    

http://AdamDempsey.net
Posts: 2395 Reputation: 53
37 / / 
Joined: Jul 2003
|
RE: [C++ Tutorial] Events and the Plus API
a Wiki sounds a great idea and im sure theres a few other coders about who help too and once my knwoledge in C++ improves i could input some (basic) code 
|
|
03-02-2005 09:55 PM |
|
 |
RaceProUK
Elite Member
    

Posts: 6073 Reputation: 57
39 / / 
Joined: Oct 2003
|
O.P. RE: [C++ Tutorial] Events and the Plus API
Are you willing to host it?
Also, with a Wiki, we can have Messenger API information available for all.
|
|
03-03-2005 09:09 AM |
|
 |
TheBlasphemer
Senior Member
   
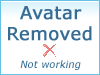
Posts: 714 Reputation: 47
36 / – / –
Joined: Mar 2004
|
RE: [C++ Tutorial] Events and the Plus API
quote: Originally posted by raceprouk
Are you willing to host it?
Also, with a Wiki, we can have Messenger API information available for all.
I can host it, I'll send you the info in two days 
First have a little lanparty today and tomorrow 
|
|
03-03-2005 09:35 AM |
|
 |
Dempsey
Scripting Contest Winner
    

http://AdamDempsey.net
Posts: 2395 Reputation: 53
37 / / 
Joined: Jul 2003
|
RE: [C++ Tutorial] Events and the Plus API
Ah TB replied before me, well if TB cant host i will, ive still got 11GB of hosting left 
|
|
03-03-2005 11:05 AM |
|
 |
|