Tic-Tac-Toe |
Author: |
Message: |
saralk
Veteran Member
    

Posts: 2598 Reputation: 38
35 / / 
Joined: Feb 2003
|
O.P. Tic-Tac-Toe
I made a game of tic-tac-toe in computing today!
Its pretty cool, you can play against a computer, although the computer is very easy to beat, there is a severe lack of interface and there are a few debug things left over.
this is the code to it:
code: program tictactoe;
{Saral Kaushik}
{$apptype console}
var
Board : array [1..3, 1..3] of char;
Turn : integer;
PlayGame : boolean;
procedure SetBoard;
var
SetLoop : integer;
SetLoop2 : integer;
begin
for SetLoop := 1 to 3 do
for SetLoop2 := 1 to 3 do
Board[SetLoop, SetLoop2] := '.';
end;
procedure DisplayBoard;
var
DisplayLoop : integer;
DisplayLoop2 : integer;
begin
writeln('1 2 3');
for DisplayLoop := 1 to 3 do
begin
for DisplayLoop2 := 1 to 3 do
write(Board[DisplayLoop, DisplayLoop2],' ');
writeln(DisplayLoop,' ');
end;
end;
function PlayerTurn(Character : char):boolean;
var
XPosition : integer;
YPosition : integer;
Move : boolean;
begin
Move := FALSE;
while Move = FALSE do
begin
write('Enter X Position: ');
readln(XPosition);
write('Enter Y Position: ');
readln(YPosition);
if Board [YPosition, XPosition] = '.' then
begin
Board [YPosition, XPosition] := Character;
Move := TRUE;
end
else
writeln('Position Taken');
end;
end;
function BoardFull():boolean;
var
FullLoop : integer;
FullLoop2 : integer;
Total : integer;
begin
Total := 0;
for FullLoop := 1 to 3 do
for FullLoop2 := 1 to 3 do
if (Board[FullLoop, FullLoop2] <> '.') then Total := Total + 1;
if (Total = 9) then BoardFull := TRUE
else BoardFull := FALSE;
end;
function IsWin(CheckFor:char):boolean;
const
WinString = '111000000000111000000000111100010001001010100100100100010010010001001001';
var
CheckLoopX : integer;
CheckLoopY : integer;
MatchCount : integer;
StringNo : integer;
begin
MatchCount := 0;
StringNo := 1;
repeat
for CheckLoopX := 1 to 3 do
for CheckLoopY := 1 to 3 do
begin
if ((Board [CheckLoopX, CheckLoopY] = CheckFor) and (WinString[StringNo] = '1')) then
MatchCount := MatchCount + 1;
//writeln (CheckLoopX, ' ',CheckLoopY,' ',Board[CheckLoopX, CheckLoopY],' ',WinString[StringNo],' ',MatchCount);
StringNo := StringNo + 1;
end;
//writeln;
if (MatchCount < 3) then MatchCount := 0;
until (MatchCount >= 3) or (StringNo >= 72);
if MatchCount >= 3 then begin
IsWin := TRUE; //writeln('Win')
end
else IsWin := FALSE;
end;
function MakeMove(Player:char):boolean;
const
MoveString = '112000000000112000000000112211000000000211000000000211121000000000121000000000121100100200010010020001001002200100100020010010002001001100200100010020010001002001100010002200010001100020001002010100001010200001020100';
var
CheckLoopX : integer;
CheckLoopY : integer;
MatchCount : integer;
StringNo : integer;
MoveX : integer;
MoveY : integer;
OtherPlayer : char;
begin
//check to see if a win is possible
MatchCount := 0;
StringNo := 1;
MoveX := 0;
MoveY := 0;
repeat
for CheckLoopX := 1 to 3 do
begin
for CheckLoopY := 1 to 3 do
begin
if (MoveString [StringNo] = '2') and (Board [CheckLoopX, CheckLoopY] = '.') then
begin
MoveX := CheckLoopX;
MoveY := CheckLoopY;
end;
if (MoveString[StringNo] = '1') and (Board [CheckLoopX, CheckLoopY] = Player) then
begin
MatchCount := MatchCount + 1;
//writeln('matched');
//readln;
end;
//writeln(StringNo,' ',Board[CheckLoopX, CheckLoopY],' ',MoveString[StringNo],' ',MatchCount);
StringNo := StringNo + 1;
end;
end;
if (MatchCount < 2) then MatchCount := 0;
//writeln;
until (((MatchCount >= 2) and (MoveX <> 0)) or (StringNo >= 216));
if ((MatchCount >= 2) and (MoveX <> 0)) then
begin
Board [MoveX, MoveY] := Player;
writeln('Win Possible Move Made');
end
else
begin
//check to see if a block is possible
MatchCount := 0;
StringNo := 1;
repeat
for CheckLoopX := 1 to 3 do
for CheckLoopY := 1 to 3 do
begin
if (MoveString [StringNo] = '2') and (Board [CheckLoopX, CheckLoopY] = '.') then
begin
MoveX := CheckLoopX;
MoveY := CheckLoopY;
end;
if (Player = 'X') then OtherPlayer := 'O'
else OtherPlayer := 'X';
if (MoveString[StringNo] = '1') and (Board [CheckLoopX, CheckLoopY] = OtherPlayer) then MatchCount := MatchCount + 1;
//writeln(StringNo,' ',Board[CheckLoopY, CheckLoopX],' ',MoveString[StringNo],' ',MatchCount);
StringNo := StringNo + 1;
end;
if (MatchCount < 2) then MatchCount := 0;
until (((MatchCount >= 2) and (MoveX <> 0)) or (StringNo >= 216));
if (MatchCount >= 2) and (MoveX <> 0) then
begin
Board [MoveX, MoveY] := Player;
writeln('Block Possible Move Made', MatchCount);
end
else
begin
repeat
randomize;
MoveX := Random(4);
MoveY := Random(4);
until (Board [MoveX, MoveY] = '.');
Board [MoveX, MoveY] := Player;
writeln('Random Move Made');
end;
end;
end;
begin
SetBoard;
DisplayBoard;
begin
Turn := 1;
PlayGame := TRUE;
repeat
if Turn = 1 then
begin
writeln('Player 1');
PlayerTurn('X');
DisplayBoard;
Turn := 0;
end
else
begin
{writeln('Player 2');
PlayerTurn('O');
DisplayBoard;
if IsWin('O') then
begin
writeln('Player 2 Wins');
PlayGame := FALSE;
end;}
MakeMove('O');
DisplayBoard;
Turn := 1;
end;
if (IsWin('X') = TRUE) then writeln('Human Wins, Well Done Human')
else if (IsWin('O') = TRUE) then writeln('Computer Wins, Better Luck Next Time Human');
until ((BoardFull = TRUE) or (PlayGame = FALSE));
end;
readln;
end.
Attachment: tictactoe.exe (18 KB)
This file has been downloaded 199 time(s).
This post was edited on 11-29-2005 at 06:35 PM by saralk.
The Artist Formerly Known As saralk
London · New York · Paris
Est. 1989
|
|
11-29-2005 03:46 PM |
|
 |
Rubber Stamp
Senior Member
   

It Was Never Random
Posts: 587 Reputation: 14
34 / / –
Joined: May 2004
|
RE: Tic-Tac-Toe
c++? its good timepass..lol
|
|
11-29-2005 03:50 PM |
|
 |
CookieRevised
Elite Member
    

Posts: 15519 Reputation: 173
– / / 
Joined: Jul 2003
Status: Away
|
RE: Tic-Tac-Toe
quote: Originally posted by Rubber Stamp
c++?
Hexview it and take a calculated guess: Borland Delphi
.-= A 'frrrrrrrituurrr' for Wacky =-.
|
|
11-29-2005 04:10 PM |
|
 |
Rubber Stamp
Senior Member
   

It Was Never Random
Posts: 587 Reputation: 14
34 / / –
Joined: May 2004
|
RE: Tic-Tac-Toe
what?? sorry i dont get you cookie.
* Rubber Stamp feels soo stupid!
|
|
11-29-2005 04:23 PM |
|
 |
CookieRevised
Elite Member
    

Posts: 15519 Reputation: 173
– / / 
Joined: Jul 2003
Status: Away
|
|
11-29-2005 04:28 PM |
|
 |
user27089
Disabled Account
Posts: 6321
Joined: Nov 2003
Status: Away
|
RE: Tic-Tac-Toe
That game is amazing!!! I keep winning ;p.
Edit:
![[Image: attachment.php?pid=571387]](http://shoutbox.menthix.net/attachment.php?pid=571387)
Attachment: I WON!.JPG (28.03 KB)
This file has been downloaded 328 time(s).
This post was edited on 11-29-2005 at 04:40 PM by user27089.
|
|
11-29-2005 04:37 PM |
|
 |
MeEtc
Patchou's look-alike
    

In the Shadow Gallery once again
Posts: 2200 Reputation: 60
38 / / 
Joined: Nov 2004
Status: Away
|
RE: Tic-Tac-Toe
cool!
If you're not too protective, and it is in Delphi, could you send the source along? I like toying with Delphi.
I might fix the look up a bit 
![[Image: sharing.png]](http://www.meetcweb.com/files/sharing.png)
I cannot hear you. There is a banana in my ear.
|
|
11-29-2005 05:55 PM |
|
 |
John Anderton
Elite Member
    
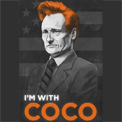
Posts: 3908 Reputation: 80
37 / / 
Joined: Nov 2004
Status: Away
|
RE: Tic-Tac-Toe
quote: Originally posted by traxor
Attachment: I WON!.JPG (28.03 KB)
This file has been downloaded 17 time(s).
This post was edited Today at 10:10 PM by traxor.
Thats how i won too  But it still asks for my input for the one remaining space 
saral .....
[
KarunAB.com]
[img]http://gamercards.exophase.com/459422.png[
/img]
|
|
11-29-2005 06:04 PM |
|
 |
Negro_Joe
Senior Member
   
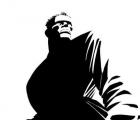
Go!Go!Go!
Posts: 692 Reputation: 21
34 / / –
Joined: Aug 2005
|
RE: Tic-Tac-Toe
lol..i enjoyed that for the 10 seconds i was playing on it... 
|
|
11-29-2005 06:09 PM |
|
 |
saralk
Veteran Member
    

Posts: 2598 Reputation: 38
35 / / 
Joined: Feb 2003
|
O.P. RE: Tic-Tac-Toe
quote: Originally posted by John Anderton
quote: Originally posted by traxor
Attachment: I WON!.JPG (28.03 KB)
This file has been downloaded 17 time(s).
This post was edited Today at 10:10 PM by traxor.
Thats how i won too But it still asks for my input for the one remaining space 
saral .....
yeah, I know about that bug, I just need to change it around a bit. I got a bit over excited and forgot that i still had that left to do.
quote: Originally posted by MeEtc
cool!
If you're not too protective, and it is in Delphi, could you send the source along? I like toying with Delphi.
I might fix the look up a bit 
i'll post the code next time i'm in school. Edit: Just realised I can access my school folder from home, see above
quote: Originally posted by CookieRevised
quote: Originally posted by Rubber Stamp
c++?
Hexview it and take a calculated guess: Borland Delphi
correct, as ever!
This post was edited on 11-29-2005 at 06:34 PM by saralk.
The Artist Formerly Known As saralk
London · New York · Paris
Est. 1989
|
|
11-29-2005 06:21 PM |
|
 |
Pages: (2):
« First
[ 1 ]
2
»
Last »
|
|