Problems with ChatWnd |
Author: |
Message: |
neocon
New Member

Posts: 2
Joined: Feb 2007
|
O.P. Problems with ChatWnd
Hello fellas, I am trying to design a simple program that will encrypt a message on input and send the encrypted through msn. Alright, it works like this so far. When you type /Crypto you enable the encryption-machine, then you type whatever you want and when you eventually want to go back to normal you type /ExitCrypto. Alright, I am new to JScript and well I've got a few problems, I managed to make it work partially, however it doesn't really send the right message. I've written this program in C and it works perfectly so the algorithm is definitely not a problem. I will be glad if you guys can be of any help, thanks a bunch in advance
code: var flag = new Boolean();
var CryptoTable = [], i = 8;
function OnEvent_Initialize(MessengerStart) {
Debug.trace("Crypto Script v0.1 started.");
parseIntoTable();
flag = false;
}
function OnEvent_Uninitialize(MessengerExit) {
Debug.trace("Crypto Script v0.1 terminated.");
}
function OnEvent_ChatWndSendMessage(ChatWnd, Message) {
if(Message == "/Crypto") {
flag = true;
return "";
}
if(Message == "/ExitCrypto") {
flag = false;
return "";
}
if(flag) {
var i = 0;
var mesg = Message;
var outString = "";
while(i < Message.length) {
if(mesg.charAt( i ) == ' ') {
outString = outString + "#";
i++;
continue;
}
for(var x = 0; x < 8; x++) {
for(var y = 0; y < 4; y++) {
if(CryptoTable[x][y] == mesg.charAt( i )) {
for(var z = 0; z < y + 1; z++) {
var send = x + 2;
outString = outString + send;
}
}
}
}
if( ((i+1) != Message.length) && (mesg.charAt(i+1) != ' ') ) {
outString = outString + "*";
}
i++;
}
ChatWnd.SendMessage(outString);
return "";
}
return Message;
}
function parseIntoTable() {
Debug.trace("parseIntoTable() called");
do
CryptoTable.push([]);
while(0 < --i);
CryptoTable[0] = ['A', 'B', 'C', -1 ];
CryptoTable[1] = ['D', 'E', 'F', -1 ];
CryptoTable[2] = ['G', 'H', 'I', -1 ];
CryptoTable[3] = ['J', 'K', 'L', -1 ];
CryptoTable[4] = ['M', 'N', 'O', -1 ];
CryptoTable[5] = ['P', 'Q', 'R', 'S'];
CryptoTable[6] = ['T', 'U', 'V', -1 ];
CryptoTable[7] = ['W', 'X', 'Y', 'Z'];
}
C function
code: void encrypt(char *mesg) {
int i = 0;
for(int x = 0; mesg[x] != '\0'; x++)
mesg[x] = toupper(mesg[x]);
while(mesg[i] != '\0') {
if(mesg[i] == ' ') {
cout << "#";
i++;
continue;
}
for(int x = 0; x < 8; x++) {
for(int y = 0; y < 4; y++) {
if(CryptoTable[x][y] == mesg[i]) {
for(int z = 0; z < y + 1; z++) {
cout << (x+2);
}
}
}
}
if((mesg[i+1] != '\0') && (mesg[i+1] != ' ') )
cout << "*";
i++;
}
}
This post was edited on 02-19-2007 at 06:51 PM by neocon.
|
|
02-19-2007 06:48 PM |
|
 |
Patchou
Messenger Plus! Creator
    
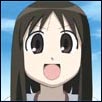
Posts: 8607 Reputation: 201
43 / / 
Joined: Apr 2002
|
RE: Problems with ChatWnd
if(Message == "/Crypto") {
flag = true;
return "";
}
.. why the return statement here? the rest of your funciton is never executed!
Also, replace the last:
ChatWnd.SendMessage(outString);
return "";
by
return outString;
|
|
02-20-2007 04:01 AM |
|
 |
vikke
Senior Member
   
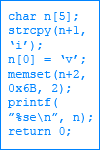
Posts: 900 Reputation: 28
31 / / 
Joined: May 2006
|
RE: RE: Problems with ChatWnd
quote: Originally posted by Patchou
if(Message == "/Crypto") {
flag = true;
return "";
}
.. why the return statement here? the rest of your funciton is never executed! 
Well.. It is executed, if the message isn't "/Crypto" or "/CryptoExit" and "Crypto" is enabled.
|
|
02-20-2007 07:57 AM |
|
 |
neocon
New Member

Posts: 2
Joined: Feb 2007
|
O.P. RE: Problems with ChatWnd
Well, I used the return statement there so that when the user types /Crypto, it will not send the message itself but just enable the encryption thing. Well it seems that the return outString; is what I needed, even though it doesn't work yet but that is because I probably made a mistake in the algorithm which I am about to correct right now. Thanks for the help
Edit: So guys, yes it works
the code here for anyone who is interested
code: var flag = new Boolean();
var CryptoTable = [], i = 8;
function OnEvent_Initialize(MessengerStart)
{
Debug.trace("Crypto Script v0.1 started.");
parseIntoTable();
flag = false;
}
function OnEvent_Uninitialize(MessengerExit)
{
Debug.trace("Crypto Script v0.1 terminated.");
}
function OnEvent_ChatWndSendMessage(ChatWnd, Message)
{
if(Message == "/Crypto") {
flag = true;
return "";
}
if(Message == "/ExitCrypto") {
flag = false;
return "";
}
if(flag) {
var i = 0;
var mesg = Message;
var outString = "";
while(i < Message.length) {
if(mesg.charAt( i ) == " ") {
outString = outString + "#";
i++;
continue;
}
for(var x = 0; x < 8; x++) {
for(var y = 0; y < 4; y++) {
if(CryptoTable[x][y] == mesg.charAt( i )) {
for(var z = 0; z < y + 1; z++) {
var send = (x + 2);
outString = outString + send;
}
}
}
}
if( ((i+1) != Message.length) && (mesg.charAt(i+1) != ' ') ) {
outString = outString + "*";
}
i++;
}
return outString;
}
return Message;
}
function parseIntoTable() {
Debug.trace("parseIntoTable() called");
do
CryptoTable.push([]);
while(0 < --i);
CryptoTable[0] = ['A', 'B', 'C', -1 ];
CryptoTable[1] = ['D', 'E', 'F', -1 ];
CryptoTable[2] = ['G', 'H', 'I', -1 ];
CryptoTable[3] = ['J', 'K', 'L', -1 ];
CryptoTable[4] = ['M', 'N', 'O', -1 ];
CryptoTable[5] = ['P', 'Q', 'R', 'S'];
CryptoTable[6] = ['T', 'U', 'V', -1 ];
CryptoTable[7] = ['W', 'X', 'Y', 'Z'];
}
This post was edited on 02-21-2007 at 01:59 AM by WDZ.
|
|
02-20-2007 05:06 PM |
|
 |
|
|