trouble with strings [C++] |
Author: |
Message: |
cooldude_i06
Full Member
  

I'm so cool I worry myself.
Posts: 272 Reputation: 9
– / / –
Joined: Sep 2003
|
O.P. trouble with strings [C++]
I'm having trouble receiving strings from a dll.
First I create a Databloc of whatever length and pass the pointer in an Interop Call. On the function side the dll receives it as:
code: int function(wchar_t* param)
Then I create a temporary string and assign it to the one function received.
code: wchar_t* test = L"Hello World";
param = test;
And then I try to read the string from Plus using Interop.ReadString but that returns nothing. I know I'm doing something really stupid just need some one to point it out. This is just a test code that I need to get running (and understand) to do something more complex.
------------------------------------
Issue 2:
It would be better for me to have the string as single byte chars. I have everything working with char* strings but I do not know how I can get Plus to read single byte ASCII strings from memory. ReadString function assigns them weird characters. This would be better for me because further on I have to read buffers from memory that consist of u_char.
THanks
CD
This post was edited on 06-08-2007 at 04:26 AM by cooldude_i06.
|
|
06-08-2007 03:40 AM |
|
 |
TazDevil
Full Member
  
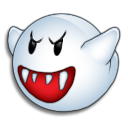
sleep(0);
Posts: 359 Reputation: 11
40 / / 
Joined: May 2004
|
RE: trouble with strings [C++]
you must appreciate the scope of the pointer creation and life time...
you create a databloc in Plus! before calling the c++ function... this pointer can exist globaly to the script.
then in your c++ module you replace a string that is only created and available on the scope of hte particular function in c++ so once the function returns the pointer *test is no longer available, as you exit the scope of the function.
instead you should write to the pointer already supplied by Plus!, the databloc, so it will be readable by Plus! after modifications... or create a new memory allocation with the c++ function that you will need to release later on, to avoid memory leaks, buildups etc...
so you use ...
int function(char* string, int len)
{
// string is the pointer to your databloc you created in plus script
// len points to the maximum space allocated in the databloc, to make sure you dont exceed the limit.
return strncpy(string, "Hello World!", len);
}
this way you write to the same pointer which is valid in plus as well.
quote: From script manual, databloc object
[string] ReadString(
[number] Offset,
[boolean,optional] ReadUnicode,
[number,optional] Size
);
you can specifically read ASCII you assigning ReadUnicode to FALSE as it is TRUE by default.
Window Manager is discontinued, for WLM try the new,
Enhancer for contact list docking, auto-hide, hide taskbar button, remove button flashing and remember keyboard layout in conversation windows
|
|
06-08-2007 07:49 AM |
|
 |
cooldude_i06
Full Member
  

I'm so cool I worry myself.
Posts: 272 Reputation: 9
– / / –
Joined: Sep 2003
|
O.P. RE: trouble with strings [C++]
Thanks TazDevil, the scope issue didn't cross my mind at all and strncpy function worked perfectly!
Now on to the second problem:
What I have is a large buffer of unsigned chars (const u_char* buffer). This buffer is created at the start and the function does not end so we don't have to worry about the scope.
What I want to do is basically read this buffer in the Plus script.
I have tried typecasting it to char and assigning it to a pointer passed by the script but that doesn't help.
code: const char* passedVariable = reinterpret_cast <const char*> (buffer);
Second I tried copying entire buffer to a Databloc allocated by the script using RtlCopyMemory, but I couldn't get the syntax correct.
I just want to be able to read this buffer into the script in the most efficient way possible. In fact I would even like to avoid copying memory. If anyone has suggestions, they would be much appreciated.
Thanks
CD
|
|
06-09-2007 08:54 AM |
|
 |
vikke
Senior Member
   
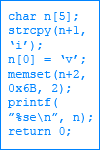
Posts: 900 Reputation: 28
31 / / 
Joined: May 2006
|
RE: trouble with strings [C++]
To read it in a Plus! script, it should be a unicode string (wchar_t).
code: const char* passedVariable = reinterpret_cast <const char*> (buffer);
That should work, but the string is still not unicode.
|
|
06-09-2007 09:07 AM |
|
 |
cooldude_i06
Full Member
  

I'm so cool I worry myself.
Posts: 272 Reputation: 9
– / / –
Joined: Sep 2003
|
O.P. RE: trouble with strings [C++]
Ok, what I would like to is copy the buffer to a DataBloc allocated by Plus. I can get the address to the start of the buffer, and I have the size of the buffer. I just cannot figure out the syntax to copy memory (RtlCopyMemory)
|
|
06-09-2007 08:29 PM |
|
 |
TazDevil
Full Member
  
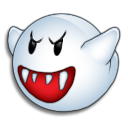
sleep(0);
Posts: 359 Reputation: 11
40 / / 
Joined: May 2004
|
RE: RE: trouble with strings [C++]
quote: Originally posted by cooldude_i06
Ok, what I would like to is copy the buffer to a DataBloc allocated by Plus. I can get the address to the start of the buffer, and I have the size of the buffer. I just cannot figure out the syntax to copy memory (RtlCopyMemory)
since you have the new string in your databloc with strncpy it copies the string from your buffer to the databloc so all you have to do is read it from the databloc in the script with ReadString() like I explained above
why you need RtlCopyMemory for?
Window Manager is discontinued, for WLM try the new,
Enhancer for contact list docking, auto-hide, hide taskbar button, remove button flashing and remember keyboard layout in conversation windows
|
|
06-09-2007 08:44 PM |
|
 |
cooldude_i06
Full Member
  

I'm so cool I worry myself.
Posts: 272 Reputation: 9
– / / –
Joined: Sep 2003
|
O.P. RE: trouble with strings [C++]
No No Taz that was issue 1, which was solved as I said before, this new issue was that I wanted to copy a buffer to a Databloc allocated by the script.
Anyways, through trial and error I got RtlCopyMemory function working. I'll post the code in case someone one day is searching for it:
code: var data = Interop.Allocate(10); //buffer size 10
Interop.Call("some.dll", "foo", data.DataPtr);
code: function foo(void* data){
RtlCopyMemory(data, &buffer[0], 10); //buffer size 10
}
Now my next issue is that ReadString function stops at the first instance of null character. The buffer however contains many null characters. The alternative is to loop and use GetAt to filter out the null characters and retrieve the rest, but if someone knows of a more efficient method please let me know.
Thanks,
CD
|
|
06-09-2007 10:29 PM |
|
 |
CookieRevised
Elite Member
    

Posts: 15519 Reputation: 173
– / / 
Joined: Jul 2003
Status: Away
|
RE: trouble with strings [C++]
The question you need to ask yourself first is what are you going todo with the data in the Plus! script.
This is highly important as this dictates how the data is to be send. eg: if you are going to show the data, the data must be in unicode.
If you are going to manipulate the data with JScript functions like Replace, Substr, etc it must be unicode too. If the data is like a picture stream or something you need to send it as real byte data (of course you can show this as a string too, but this requires some additional converting with the proper APIs (never simply add or remove null bytes when you want to convert from or to unicode!!!)).
But I assume you are at least going to manipulate the recieved data with Jscript functions. So send it as unicode...
But for byte arrays you can not use C-style strings (null terminated strings), you need to use BSTRs, which can contain null bytes since the length of the string is not defined by the first occurance of a null byte, but by the length indicator in front of the string.
BSTRs are unicode. But don't simply add null bytes to your original data bytes to make them unicode. That will not work and will render wrong results. You need to use proper ascii to unicode convertion.
PS: I hope I make some sense here, it is very late (or better early) here
PS2: all these problems you have for your sniffer (multithreading, infinitate loops, strings/data sending) can all easly be avoided if you use/make an AciveX DLL though 
This post was edited on 06-10-2007 at 03:12 AM by CookieRevised.
.-= A 'frrrrrrrituurrr' for Wacky =-.
|
|
06-10-2007 03:06 AM |
|
 |
cooldude_i06
Full Member
  

I'm so cool I worry myself.
Posts: 272 Reputation: 9
– / / –
Joined: Sep 2003
|
O.P. RE: RE: trouble with strings [C++]
quote: Originally posted by CookieRevisedPS2: all these problems you have for your sniffer (multithreading, infinitate loops, strings/data sending) can all easly be avoided if you use/make an AciveX DLL though 
Yeah but ActiveX Dll have their own problems too, like not being freed on script termination and rendering the script irremovable. That and mainly the Winpcap library does not support VB, and the VB wrapper for winpcap which is called PacketX only supports the previous version of Winpcap which does not support Vista. Lol, so yeah this is my only option.
Anyways I think i will stick to using GetAt to convert one char at a time within the script since I have reduced the amount of times I rely on it anyways. Thanks for your help.
This post was edited on 06-10-2007 at 07:07 AM by cooldude_i06.
|
|
06-10-2007 07:06 AM |
|
 |
TheBlasphemer
Senior Member
   
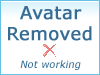
Posts: 714 Reputation: 47
36 / – / –
Joined: Mar 2004
|
RE: trouble with strings [C++]
Ok, my two cents, how about reading up on the C or C++ language before just trying to bash in code?
RtlCopyMemory is *not* the proper way to copy a string, &buffer[0] is useless, just hard-coding the value 10 is nonsense too, as this indicates the length of the data to be copied, not the size of the destination block :/
Go read some C tutorials, especially focus on pointers and arrays, and after that try to write a proper program. If you continue just trying like this, you will end up with a DLL full of buffer overflows, faulty pointers, messed up stacks, and it will not be stable and if used with data retrieved from the net, it will probably be a huge security risk too...
|
|
06-10-2007 12:05 PM |
|
 |
Pages: (2):
« First
[ 1 ]
2
»
Last »
|
|