Random numbers, prevent from being used more than once |
Author: |
Message: |
stu
Junior Member
 
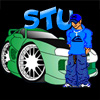
Stu
Posts: 82 Reputation: 1
38 / / 
Joined: Sep 2004
|
O.P. Random numbers, prevent from being used more than once
Ok, so I got bored today and was thinking what else I could add to a script that I have been working on that displays a random lyric. What I want to do, is make it so that the random number that is generated is not repeated within a certain amount of number generations. So for example, the numer 7 is generated, and then that number cant be used again until 10 different numbers have been used. Something like that.
What I am looking for are ideas to do that.. I looked online, and all I could find was ways to prevent using the same number twice in a row.. But I am looking for a way to prevent the same lyric being used until, lets say 10, different lyrics have been used. I dont like using the same lyric every other or every 4 times or something, and that has happened.
Anyways, does anyone have any ideas how to go about doing this? I was thinking of storing the random number into an array and then comparing the new random number against the array to make sure they are different.. but I couldnt find any way to make that work. Any new ideas or help would be great, thanks
|
|
07-03-2007 10:14 PM |
|
 |
pollolibredegrasa
Full Member
  

formerly fatfreechicken
Posts: 483 Reputation: 34
35 / / 
Joined: May 2005
|
RE: Random numbers, prevent from being used more than once
Hmm, there are probably better ways to do this, but you could possibly do this using a multidimensional array...
I'm not sure how to explain but in my head it makes sense. Basically create an array for all the used lyrics, in which each item is also an array containing the 1) lyric number and 2) number of times a lyric has been generated since that one.
So say you generate lyric number 8, the used lyrics array would contain [8,0]
Then every time you generate another lyric, add it to the usedlyrics array and increment the second array item for all the previously generated ones until they are 10, at which point you can remove the item from the usedlyrics array.
Thus if you generate another lyric (say, number 3), your usedlyrics array would contain [8,1],[3,0].
Then another lyric (say number 9): [8,2],[3,1],[0,9] etc.
Obviously you have to make sure the lyric generated is not already in the usedlyrics array before adding it though =p
Sorry if I'm not making myself clear, I'm tired and not feeling great but it sounded good in my head 
;p
Vaccy is my thin twin! ![[Image: chickennana.gif]](http://shoutbox.menthix.net/images/smilies/chickennana.gif)
|
|
07-03-2007 10:33 PM |
|
 |
foaly
Senior Member
   
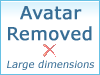
Posts: 718 Reputation: 20
38 / / 
Joined: Jul 2006
|
RE: Random numbers, prevent from being used more than once
the easiest thing to do would be an array and a function like:
function a{
int i is random;
for(int j=0,j<array.length;j++){
if(i==array[j]){
return a();
}
}
array[count]=i;
count++;
count=count%9;
return i;
}
something like that...
|
|
07-03-2007 10:34 PM |
|
 |
roflmao456
Skinning Contest Winner
   

Posts: 955 Reputation: 24
29 / / 
Joined: Nov 2006
Status: Away
|
RE: Random numbers, prevent from being used more than once
Create this at the top of your script:
code: var Array = new Array();
Then put this code to where the random thing is:
code: var random = Math.floor(Math.random()*10 /* 10 lyrics.. */);
for(i=0;i<Array.length;i++){
if(Array[random] != i){
Array[random] = i;
return;
}
}
i'm not sure if that will work..
[quote]
Ultimatess6: What a noob mod
|
|
07-04-2007 03:15 AM |
|
 |
Volv
Skinning Contest Winner
    

Posts: 1233 Reputation: 31
34 / / 
Joined: Oct 2004
|
RE: Random numbers, prevent from being used more than once
I think foaly's is the simplest working code.
Although I think instead of:
count=count%9
You should replace it with:
count= (count==9 ? 0:count);
Because you don't want to have unnecessarily large integers being stored in memory.
EDIT: actually ignore this, foaly's does exactly the same  My mistake.
This post was edited on 07-04-2007 at 05:45 PM by Volv.
|
|
07-04-2007 06:01 AM |
|
 |
markee
Veteran Member
    

Posts: 1621 Reputation: 50
36 / / 
Joined: Jan 2006
|
RE: Random numbers, prevent from being used more than once
why use count at all when j will give you the same result?
your code should just be this:
code: var lyricsArray = new Array("Come fly with me, lets fly, lets fly away","Can't buy me love","Yesterday, all my troubles seemed so far away");
var selectedArray = new Array();
function chooseRandomLyric(){
var i = Math.round(Math.random()*(lyricsArray.length-1));
var j;
Inner:
for(j=0;j<selectedArray.length;j++){
if(i==selectedArray[j]){
i=Math.round(Math.random()*lyricsArray.length);
continue Inner;
}
}
if(selectedArray.length === 10){
selectedArray.pop;
}
selectedArray.concat(i,selectedArray);
return i;
}
I did label a function which a lot of people are probably unfamiliar with, but it makes it a little more resource friendly as you can be calling the same function hundreds of times (there is a chance that it will never give you an answer and freeze your computer too.... but this is very limited, but more probable when you have a lot of lyrics and have gone through most of them). And I also added an if statement at the end to make sure that there is no case where there are no lyrics left... which would leave you with an infinite loop too.
Furthermore, dynamic functions are a very wise idea and that is why I have made the random number based upon the array of lyrics rather than you having to do that manually when you update it. I hope you enjoy.
EDIT: updated code due to Volv's post below
This post was edited on 07-04-2007 at 02:41 PM by markee.
|
|
07-04-2007 02:17 PM |
|
 |
Volv
Skinning Contest Winner
    

Posts: 1233 Reputation: 31
34 / / 
Joined: Oct 2004
|
RE: Random numbers, prevent from being used more than once
markee, that code isn't very elegant and could be more optimised, but it also doesn't solve the initial problem =/
quote: Originally posted by markee
why use count at all when j will give you the same result?
'count' provides a means of allowing the lyric to be re-used after 10 different lyrics have been used as was requested:
quote: Originally posted by stu
But I am looking for a way to prevent the same lyric being used until, lets say 10, different lyrics have been used. ...
So for example, the numer 7 is generated, and then that number cant be used again until 10 different numbers have been used. Something like that.
This post was edited on 07-04-2007 at 02:32 PM by Volv.
|
|
07-04-2007 02:31 PM |
|
 |
markee
Veteran Member
    

Posts: 1621 Reputation: 50
36 / / 
Joined: Jan 2006
|
RE: Random numbers, prevent from being used more than once
quote: Originally posted by Volv
'count' provides a means of allowing the lyric to be re-used after 10 different lyrics have been used as was requested:
quote riginally posted by stu
But I am looking for a way to prevent the same lyric being used until, lets say 10, different lyrics have been used. I dont like using the same lyric every other or every 4 times or something, and that has happened.
well in that case then I just need to use a .pop on my selectedArray if the length is 10 and add the new one on.... not overly difficult. I'll edit my code above 
|
|
07-04-2007 02:35 PM |
|
 |
foaly
Senior Member
   
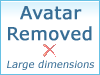
Posts: 718 Reputation: 20
38 / / 
Joined: Jul 2006
|
RE: Random numbers, prevent from being used more than once
quote: Originally posted by markee
code: var lyricsArray = new Array("Come fly with me, lets fly, lets fly away","Can't buy me love","Yesterday, all my troubles seemed so far away");
var selectedArray = new Array();
function chooseRandomLyric(){
var i = Math.round(Math.random()*(lyricsArray.length-1));
var j;
Inner:
for(j=0;j<selectedArray.length;j++){
if(i==selectedArray[j]){
i=Math.round(Math.random()*lyricsArray.length);
continue Inner;
}
}
if(selectedArray.length === 10){
selectedArray.pop;
}
selectedArray.concat(i,selectedArray);
return i;
}
this option maybe nicer...
but it might be easier to use a function you understand...
and I doubt the thread starter understands how exactly your function works... 
|
|
07-04-2007 04:00 PM |
|
 |
stu
Junior Member
 
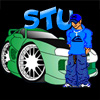
Stu
Posts: 82 Reputation: 1
38 / / 
Joined: Sep 2004
|
O.P. RE: Random numbers, prevent from being used more than once
Wow, thanks for the responses guys, didnt expect so many.. I'll give your suggestions all a try today and see what I come up with. A lot of it, I dont even understand though, like markee's code, lol. But I will give it a try anyways.
foaly, in your code, what does "count=count%9;" that line mean? Never seen that before..
Also, Im going to attach a copy of my script on here as it is right now if you want to take a look at it to better understand what I am doing. I took out the lyrics to cut down on filesize, but if anyone is interested in seeing them as well, just let me know.
Probably later on tonight I'll get back to you about what my results were, thanks again for the suggestions
Edit: Replaced file with current working version, with this implemented in it, and all lyrics I currently have for anyone who is interested.
Attachment: Jeremy's Random Song Lyric.zip (29.12 KB)
This file has been downloaded 86 time(s).
This post was edited on 07-27-2007 at 03:25 PM by stu.
|
|
07-04-2007 04:50 PM |
|
 |
Pages: (4):
« First
[ 1 ]
2
3
4
»
Last »
|
|
|