PHP Help, file upload |
Author: |
Message: |
matty
Scripting Guru
    
Posts: 8336 Reputation: 109
39 / / 
Joined: Dec 2002
Status: Away
|
O.P. PHP Help, file upload
Hey everyone, I have been trying to write a File Upload script for a site I am doing but I can't seem to find a working script.
If anyone could help me out that would be great. I have even tried ones on PSCode but they don't work. Here is the infor for the server that it will be on.
![[Image: attachment.php?pid=371412]](http://shoutbox.menthix.net/attachment.php?pid=371412)
Attachment: serverinfo.gif (4.55 KB)
This file has been downloaded 461 time(s).
|
|
01-30-2005 10:14 PM |
|
 |
KeyStorm
Elite Member
    

Inn-sewer-ants-pollie-sea
Posts: 2156 Reputation: 45
38 / / –
Joined: Jan 2003
|
RE: PHP Help, file upload
you just need a <input type="file"/ name="myFile"> input field and then an autoglobal array $_FILES['myFile'] will be available. This array has a second dimension with following keys:
name - the name of the uploaded file with extension
type - the filetype ( mime type)
size - amount of bytes of the file
tmp_name - absolute path to the temporary file (its name is random)
The rest (storing to a folder, uploading to a database, showing the file) goes to your imagination.
If you need some more help, just tell us.
|
|
01-30-2005 10:22 PM |
|
 |
L. Coyote
Senior Member
   
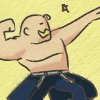
Captain Obvious
Posts: 981 Reputation: 49
38 / / 
Joined: Aug 2004
Status: Away
|
RE: PHP Help, file upload
An example of a correct form:
<form enctype="multipart/form-data" action="_URL_" method="post">
It's very important that you put that bolded part.  Else, it won't work.
More info here.
Hack, hack, hack!
Finally became a Systems Analyst!
|
|
01-30-2005 10:58 PM |
|
 |
segosa
Community's Choice
    
Posts: 1407 Reputation: 92
Joined: Feb 2003
|
RE: PHP Help, file upload
I made a file upload script the other day, it's about 100 lines. If you want me to send you it contact me on MSN Messenger, Matty.
The previous sentence is false. The following sentence is true.
|
|
01-31-2005 06:28 AM |
|
 |
megamuff
Full Member
  
Posts: 128 Reputation: -12
36 / – / –
Joined: Apr 2003
|
RE: PHP Help, file upload
code: <?php
if ($HTTP_POST_VARS['submit']) {
print_r($HTTP_POST_FILES);
if (!is_uploaded_file($HTTP_POST_FILES['file']['tmp_name'])) {
$error = "You did not upload a file!";
unlink($HTTP_POST_FILES['file']['tmp_name']);
// assign error message, remove uploaded file, redisplay form.
} else {
//a file was uploaded
$maxfilesize=125829120;
if ($HTTP_POST_FILES['file']['size'] > $maxfilesize) {
$error = "file is too large";
unlink($HTTP_POST_FILES['file']['tmp_name']);
// assign error message, remove uploaded file, redisplay form.
} else {
if ($HTTP_POST_FILES['file']['type'] != "image/gif" AND $HTTP_POST_FILES['file']['type'] != "image/pjpeg" AND $HTTP_POST_FILES['file']['type'] != "image/bmp" AND $HTTP_POST_FILES['file']['type'] != "video/mpeg" AND $HTTP_POST_FILES['file']['type'] != "video/quicktime" AND $HTTP_POST_FILES['file']['type'] != "video/x-msvideo" AND $HTTP_POST_FILES['file']['type'] != "image/png" AND $HTTP_POST_FILES['file']['type'] != "application/x-shockwave-flash") {
$error = "This file type is not allowed";
unlink($HTTP_POST_FILES['file']['tmp_name']);
// assign error message, remove uploaded file, redisplay form.
} else {
//File has passed all validation, copy it to the final destination and remove the temporary file:
copy($HTTP_POST_FILES['file']['tmp_name'],"up/".$HTTP_POST_FILES['file']['name']);
unlink($HTTP_POST_FILES['file']['tmp_name']);
print "File has been successfully uploaded!";
exit;
}
}
}
}
?>
<html>
<head>
<title>File Uploader</title>
</head>
<center>
<form action="<?=$PHP_SELF?>" method="post" enctype="multipart/form-data">
<?=$error?>
<br>if a file name has spaces in it, rename the file and take out the spaces before you upload it!!<br>
Choose a file to upload br>
<input type="file" name="file"><br>
<input type="submit" name="submit" value="submit">
</form>
</center>
</body>
</html>
|
|
02-01-2005 04:02 PM |
|
 |
segosa
Community's Choice
    
Posts: 1407 Reputation: 92
Joined: Feb 2003
|
RE: PHP Help, file upload
He already got the code, I sent him my script
code: if ($HTTP_POST_FILES['file']['type'] != "image/gif" AND $HTTP_POST_FILES['file']['type'] != "image/pjpeg" AND $HTTP_POST_FILES['file']['type'] != "image/bmp" AND $HTTP_POST_FILES['file']['type'] != "video/mpeg" AND $HTTP_POST_FILES['file']['type'] != "video/quicktime" AND $HTTP_POST_FILES['file']['type'] != "video/x-msvideo" AND $HTTP_POST_FILES['file']['type'] != "image/png" AND $HTTP_POST_FILES['file']['type'] != "application/x-shockwave-flash") {
Talk about inefficient 
The previous sentence is false. The following sentence is true.
|
|
02-01-2005 09:44 PM |
|
 |
megamuff
Full Member
  
Posts: 128 Reputation: -12
36 / – / –
Joined: Apr 2003
|
RE: PHP Help, file upload
quote: Originally posted by Segosa
He already got the code, I sent him my script 
code: if ($HTTP_POST_FILES['file']['type'] != "image/gif" AND $HTTP_POST_FILES['file']['type'] != "image/pjpeg" AND $HTTP_POST_FILES['file']['type'] != "image/bmp" AND $HTTP_POST_FILES['file']['type'] != "video/mpeg" AND $HTTP_POST_FILES['file']['type'] != "video/quicktime" AND $HTTP_POST_FILES['file']['type'] != "video/x-msvideo" AND $HTTP_POST_FILES['file']['type'] != "image/png" AND $HTTP_POST_FILES['file']['type'] != "application/x-shockwave-flash") {
Talk about inefficient 
got a better way to allow only those file types through?
|
|
02-02-2005 03:03 AM |
|
 |
WDZ
Former Admin
    

Posts: 7106 Reputation: 107
– / / 
Joined: Mar 2002
|
RE: PHP Help, file upload
I'd probably do something like this...
code: $types = array("image/gif", "image/pjpeg", "image/bmp", "video/mpeg", "video/x-msvideo", "image/png", "application/x-shockwave-flash");
if(in_array($HTTP_POST_FILES['file']['type'], $types)) {

|
|
02-02-2005 03:55 AM |
|
 |
L. Coyote
Senior Member
   
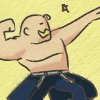
Captain Obvious
Posts: 981 Reputation: 49
38 / / 
Joined: Aug 2004
Status: Away
|
RE: PHP Help, file upload
quote: Originally posted by WDZ
I'd probably do something like this...
code: $types = array("image/gif", "image/pjpeg", "image/bmp", "video/mpeg", "video/x-msvideo", "image/png", "application/x-shockwave-flash");
if(in_array($HTTP_POST_FILES['file']['type'], $types)) {

Isn't it better to use $_FILES? 
Hack, hack, hack!
Finally became a Systems Analyst!
|
|
02-02-2005 04:06 AM |
|
 |
WDZ
Former Admin
    

Posts: 7106 Reputation: 107
– / / 
Joined: Mar 2002
|
RE: PHP Help, file upload
quote: Originally posted by Leo
Isn't it better to use $_FILES? 
Yeah, unless your version of PHP is ancient. I didn't use it because the original code didn't use it. 
|
|
02-02-2005 04:09 AM |
|
 |
Pages: (2):
« First
[ 1 ]
2
»
Last »
|
|