[help] Windows widths and heights... |
Author: |
Message: |
whiz
Senior Member
   
Posts: 568 Reputation: 8
– / – / 
Joined: Nov 2008
|
O.P. [help] Windows widths and heights...
I want to make a more visual method of creating windows in Interface Writer, and I need to know if I can get the width and height of a PlusWnd object. I'm guessing that I would need to register the WM_SIZE notification on the window, and also that I would need to use Interop, but what function?
And also, can I set the width and height of the window?
Edit: is this on the right lines?
This post was edited on 01-07-2010 at 06:12 PM by whiz.
|
|
01-06-2010 06:15 PM |
|
 |
Mnjul
forum super mod
     
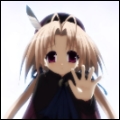
plz wub me
Posts: 5396 Reputation: 58
– / / 
Joined: Nov 2002
Status: Away
|
RE: [help] Windows widths and heights...
You can also use MoveWindow instead of SetWindowPos.
As for retrieving the window's dimension, use GetWindowRect (if there's no need to get notified every time the dimension is changed)
|
|
01-06-2010 06:25 PM |
|
 |
Spunky
Former Super Mod
    

Posts: 3658 Reputation: 61
35 / / 
Joined: Aug 2006
|
RE: [help] Windows widths and heights...
quote: Originally posted by Mnjul
if there's no need to get notified every time the dimension is changed
I would imagine he would so that he could update the xml to reflect the new size
<Eljay> "Problems encountered: shit blew up" 
|
|
01-06-2010 06:26 PM |
|
 |
Mnjul
forum super mod
     
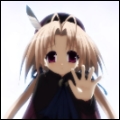
plz wub me
Posts: 5396 Reputation: 58
– / / 
Joined: Nov 2002
Status: Away
|
RE: [help] Windows widths and heights...
quote: Originally posted by Spunky
quote: Originally posted by Mnjul
if there's no need to get notified every time the dimension is changed
I would imagine he would so that he could update the xml to reflect the new size
Well, then, WM_SIZE is the way to go. Anyway its lParam encompasses the window dimension values so no need to GetWindowRect if you listen to WM_SIZE.
|
|
01-06-2010 06:28 PM |
|
 |
whiz
Senior Member
   
Posts: 568 Reputation: 8
– / – / 
Joined: Nov 2008
|
O.P. RE: [help] Windows widths and heights...
quote: Originally posted by Spunky
quote: Originally posted by Mnjul
if there's no need to get notified every time the dimension is changed
I would imagine he would so that he could update the xml to reflect the new size
Correct. I'm making a window designer that uses a blank window, with a title text box, minimize/maximize/close checkboxes and so on, as well as two text boxes that reflect the current size of the window.
Javascript code:
var WndTest = MsgPlus.CreateWnd("Interfaces\\Test.xml", "WndTest", 0);
WndTest.RegisterMessageNotification(0x5, true);
function OnWndTestEvent_MessageNotification(PlusWnd, Message, wParam, lParam)
{
if (Message === 0x5)
{
WndTest.SetControlText("EdtWidth", "");
WndTest.SetControlText("EdtWidth", "");
}
}
The problem is... the lParam contains a single, long, number. How can I separate this into the width and height?
Example: start size equates to "19661199".
This post was edited on 01-06-2010 at 06:34 PM by whiz.
|
|
01-06-2010 06:33 PM |
|
 |
Matti
Elite Member
    

Script Developer and Helper
Posts: 1646 Reputation: 39
31 / / 
Joined: Apr 2004
|
RE: [help] Windows widths and heights...
quote: Originally posted by MSDN
lParam
The low-order word of lParam specifies the new width of the client area.
The high-order word of lParam specifies the new height of the client area.
This is where bitwise operations come in handy. To get the low-order word, you need to get the first word from the number. A word is an unsigned number of 2 bytes or 16 bits. The maximum word value is thus 1111 1111 1111 1111 in binary or 65,535 in decimal or FFFF in hexadecimal. We can now use the & operator to do a bitwise AND operation. The AND operator takes two bits and returns 1 if both bits are 1, otherwise it gives 0. Applying this bit by bit on lParam and 0xFFFF, you'll get 0 for all bits further than bit 16 and bits 1 to 16 will give you the original bits from lParam in that position. This gives you the low-order word.
Javascript code:
var width = lParam & 0xFFFF;
For the height, we need to get the other 16 bits. This can be done by shifting the bits of lParam 16 bit positions to the right using the bitwise right-shift operator >>. For example: bit 20 will be shifted to bit 4 for example and bits 1 to 16 will be discarded. This gives you the high-order word.
Javascript code:
var height = (lParam >> 16);
If you want to be really sure that you're only getting 16 bits, you can do an & 0xFFFF operation on the result to discard any bits after bit 32. This won't be necessary for WM_SIZE since Windows promises you to only give you 32 bits.
Built-in operators are always lots faster than another call to GetWindowRect, so you better use them! 
This post was edited on 01-06-2010 at 06:51 PM by Matti.
|
|
01-06-2010 06:51 PM |
|
 |
whiz
Senior Member
   
Posts: 568 Reputation: 8
– / – / 
Joined: Nov 2008
|
O.P. RE: [help] Windows widths and heights...
Ok, that works great!
Next question: can I set the width and height of the window? I've tried this:
Javascript code:
Interop.Call("user32.dll", "SetWindowPos", WndTest, null, 0, 0, 300, 200, SWP_NOMOVE | SWP_NOZORDER);
But nothing happens. No error, just nothing.
|
|
01-06-2010 07:09 PM |
|
 |
Spunky
Former Super Mod
    

Posts: 3658 Reputation: 61
35 / / 
Joined: Aug 2006
|
RE: [help] Windows widths and heights...
You need to pass the handle to WndTest, not the object, don't you?
EDIT: Well I mean from WndTest to the function... before someone says something 
This post was edited on 01-06-2010 at 07:16 PM by Spunky.
<Eljay> "Problems encountered: shit blew up" 
|
|
01-06-2010 07:15 PM |
|
 |
whiz
Senior Member
   
Posts: 568 Reputation: 8
– / – / 
Joined: Nov 2008
|
O.P. RE: [help] Windows widths and heights...
True.  Ok, but now I have another problem.
Javascript code:
var SWP_NOMOVE = 2;
var SWP_NOZORDER = 4;
var WndTest = MsgPlus.CreateWnd("Interfaces\\Test.xml", "WndTest", 0);
WndTest.RegisterMessageNotification(0x5, true);
WndTest.SetControlText("EdtWidth", 400);
WndTest.SetControlText("EdtHeight", 300);
function OnWndTestEvent_MessageNotification(PlusWnd, Message, wParam, lParam)
{
if (Message === 0x5)
{
WndTest.SetControlText("EdtWidth", (lParam & 0xFFFF));
WndTest.SetControlText("EdtHeight", (lParam >> 16));
}
}
function OnWndTestEvent_CtrlClicked(PlusWnd, ControlId)
{
if (ControlId == "BtnSize")
{
Interop.Call("user32.dll", "SetWindowPos", WndTest.Handle, null, 0, 0, WndTest.GetControlText("EdtWidth"), WndTest.GetControlText("EdtHeight"), SWP_NOMOVE | SWP_NOZORDER);
}
}
That's what I have so far. But by clicking the size button after typing in, say, 300x200, I end up with an infinitely sized window. Am I missing something?
Edit: never mind, found it. Forgot parseInt().
Although, it doesn't actually size it right. 400x300 comes out as 394x268. How come?
This post was edited on 01-06-2010 at 07:23 PM by whiz.
|
|
01-06-2010 07:21 PM |
|
 |
Spunky
Former Super Mod
    

Posts: 3658 Reputation: 61
35 / / 
Joined: Aug 2006
|
RE: [help] Windows widths and heights...
Have you tried Tracing the EdtWidth and EdtHeight values? I've got a feeling it might not be reading them right. Don't know, but it might have something to do with the type conversion, although I would have imagined it would convert to an integer ok.
EDIT: parseInt... Does that mean I was right (even if I was late  )
I think the missing pixels in the size may be something to do with the window borders possibly? 32 pixels for a top border seems about right to be honest
This post was edited on 01-06-2010 at 07:28 PM by Spunky.
<Eljay> "Problems encountered: shit blew up" 
|
|
01-06-2010 07:26 PM |
|
 |
Pages: (3):
« First
[ 1 ]
2
3
»
Last »
|
|